In this lesson we want to learn How to Use Python Time Module ? first of all let’s talk about Python Time Module.
What is Time Module in Python?
time module in provides functions to work with time related values and operations. some of the common tasks you can perform using the time module include:
Getting the current time: You can use time function to get the current time in seconds since the epoch (the starting point of time in computing).
1 2 3 |
import time current_time = time.time() print(current_time) |
Converting time to readable format: You can use the gmtime and strftime functions to convert a time value to human readable format.
1 2 3 4 5 |
import time current_time = time.time() readable_time = time.gmtime(current_time) formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", readable_time) print(formatted_time) |
Run the code and this will be the result
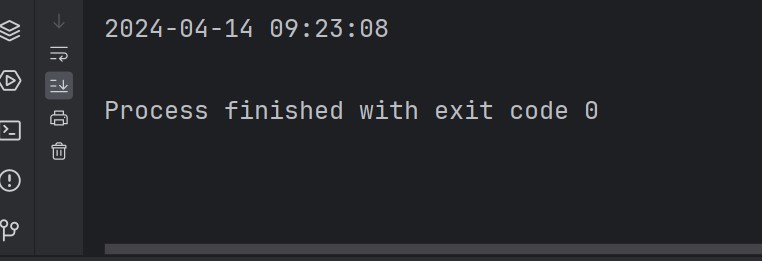
Sleeping for a specific amount of time: You can use the sleep function to pause the execution of your program for a specified amount of time.
1 2 3 4 |
import time print("Start") time.sleep(5) print("End") |
Measuring the elapsed time: You can use the time function to measure the elapsed time between two points in your program.
1 2 3 4 5 6 |
import time start_time = time.time() # Perform some operation end_time = time.time() elapsed_time = end_time - start_time print(elapsed_time) |
These are some of the basic uses of the time module in Python. You can find more information about the time module in the official Python documentation.
These are some additional details about the time module with examples:
- Converting local time to UTC with Python: mktime function can be used to convert local time expressed as struct_time to time expressed as a timestamp, assuming the local time is in UTC.
1 2 3 4 5 6 |
import time # convert local time to timestamp local_time = time.localtime() timestamp = time.mktime(local_time) print(timestamp) |
- Formatting time: strftime function can be used to format the time, and the strptime function can be used to parse string representation of the time and return a struct_time object.
1 2 3 4 5 6 7 8 9 10 11 |
import time # format time readable_time = time.gmtime() formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", readable_time) print(formatted_time) # parse time string time_string = "2022-06-24 11:00:00" parsed_time = time.strptime(time_string, "%Y-%m-%d %H:%M:%S") print(parsed_time) |
- Retrieving system timezone in Python: The tzname function returns a tuple containing the names of the local time zone and the daylight saving time zone.
1 2 3 4 |
import time timezone = time.tzname print(timezone) |
FAQs:
How do I use the time library in Python?
You can use time library in Python to work with time-related functions, such as measuring time durations, formatting dates and times, and pausing program execution. This is a basic example of how to import and use the time library:
1 2 3 4 5 6 7 8 9 |
import time # Get the current time current_time = time.time() print("Current time:", current_time) # Pause the program execution for 2 seconds time.sleep(2) print("Two seconds have passed") |
How do you use time code in Python?
In Python, you can use time module to work with time-related functionality. This includes getting the current time, formatting dates and times, measuring time durations and pausing program execution. This is a basic example:
1 2 3 4 5 6 7 8 9 |
import time # Get the current time current_time = time.time() print("Current time:", current_time) # Pause the program execution for 2 seconds time.sleep(2) print("Two seconds have passed") |
How to install Python time module?
time module is a standard library in Python, so you don’t need to install it separately. It’s included with every Python installation by default.
How do you enter time in Python?
In Python, you can get the current time using the time.time() function, and it returns the number of seconds since the epoch (January 1, 1970). You can also use other functions in the time module to format dates and times, measure time durations, and pause program execution. For example:
1 2 3 4 5 |
import time # Get the current time current_time = time.time() print("Current time:", current_time) |
Subscribe and Get Free Video Courses & Articles in your Email