In this lesson we want to learn How to List AWS IAM Users with Python & Boto3, as we already mentioned that AWS IAM enables you to manage access to AWS services securely. some times you need to list all users programmatically using Python & Boto3.
Make sure that you have already read these two article because they are related to this article.
- How to install Boto3 and AWS CLI for Python
- How to Configure AWS CLI to Use Boto3
- How to Create AWS IAM User with Python & Boto3
Prerequisites
Before we begin, ensure you have the following prerequisites:
- Python installed on your system
- Boto3 library installed (pip install boto3)
- AWS credentials configured on your system (either through AWS CLI or environment variables)
How to List AWS IAM Users with Python & Boto3
Step 1: Import Boto3
First, import the Boto3 library in your Python script:
1 |
import boto3 |
Step 2: Initialize Boto3 IAM Client
Next, we need to initialize the Boto3 IAM client, and it allows us to interact with AWS IAM:
1 |
iam = boto3.client('iam') |
Step 3: List IAM Users
Now, you can use the list_users method to retrieve a list of IAM users:
1 2 3 4 5 6 7 8 9 |
response = iam.list_users() users = response['Users'] for user in users: print("Username:", user['UserName']) print("ARN:", user['Arn']) print("UserID:", user['UserId']) print("Created On:", user['CreateDate'].strftime('%Y-%m-%d %H:%M:%S')) print() |
This code will fetch a list of IAM users and print details such as username, ARN (Amazon Resource Name), user ID, and creation date for each user.
This is the complete code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import boto3 # Initialize Boto3 IAM client iam = boto3.client('iam') # List IAM Users response = iam.list_users() users = response['Users'] for user in users: print("Username:", user['UserName']) print("ARN:", user['Arn']) print("UserID:", user['UserId']) print("Created On:", user['CreateDate'].strftime('%Y-%m-%d %H:%M:%S')) print() |
Run the code and this will be the result
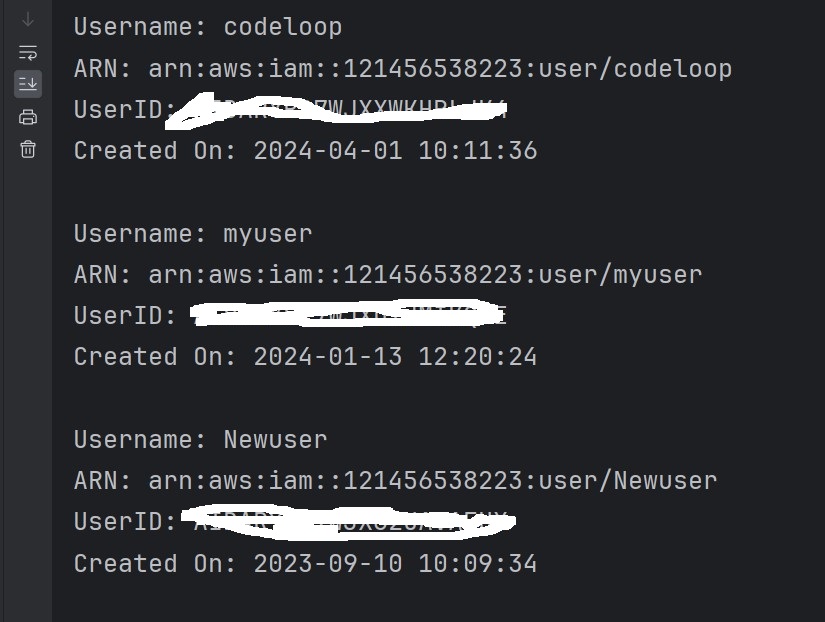
FAQS
Q: What is AWS IAM, and why would I want to list IAM users programmatically?
A: AWS IAM allows you to manage access to AWS services securely. Listing IAM users programmatically enables you to automate user management tasks, get insights into your IAM users, and integrate user management into your workflows.
Q: What is Boto3, and how does it relate to AWS IAM?
A: Boto3 is the official AWS SDK for Python, and it provides Pythonic interface to interact with AWS services, including IAM. With Boto3, you can programmatically list IAM users, create users, update permissions and perform other IAM user management tasks.
Q: How do I install Boto3 for Python?
A: Boto3 can be installed using pip, You can install Boto3 with the following command (pip install boto3).
Q: What permissions do I need to list IAM users using Boto3?
A: To list IAM users using Boto3, you need appropriate permissions granted by an IAM user, group, or role inside your AWS account. Typically, you need permissions for the iam:ListUsers action.
Subscribe and Get Free Video Courses & Articles in your Email