In this PyQt5 article i want to show you How To Export File As PDF In PyQt5, so Exporting File As PDF In PyQt5 is so simple and easy. also we are using some codes from the previous articles, in this article we will use QPrinter from PyQt5.
What is PyQt5 QPrinter ?
PyQt5 QPrinter is a class in the PyQt5 library that allows you to create and manage printer objects, which can be used to print documents or to generate PDF files. QPrinter class provides a way to specify different printing options such as the printer’s output format, the page layout and the number of copies to print.
with QPrinter, you can set up a printer object and then use it to print documents from your PyQt5 application. you can choose the printer to use, set the paper size and orientation and specify the margins for the printed pages. you can also specify whether to print in color or black and white, and whether to use a specific resolution.
In addition to printing documents, QPrinter also allows you to create PDF files from your PyQt5 application. you can use QPrinter to generate PDF file from your application’s data, and then save the file to disk or send it to a remote server.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Psyide2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
First we need some imports.
1 2 3 4 5 6 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, QTextEdit, QFontDialog, QColorDialog, QFileDialog import sys from PyQt5.QtGui import QIcon from PyQt5.QtPrintSupport import QPrintDialog, QPrinter, QPrintPreviewDialog from PyQt5.Qt import QFileInfo |
After that iam going to create my Window class that extends from QMainWindow class and in that class we create a method of CreateMenu() and in that class we add our menu requirements and methods that we have learned in the previous article. in this article we just focus that How To Export File As PDF In PyQt5.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 |
class Window(QMainWindow): def __init__(self): super().__init__() self.title = "PyQt5 QToolbar" self.top = 200 self.left = 500 self.width = 680 self.height = 480 self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) self.createEditor() self.CreateMenu() self.show() def CreateMenu(self): mainMenu = self.menuBar() fileMenu = mainMenu.addMenu('File') editMenu = mainMenu.addMenu('Edit') viewMenu = mainMenu.addMenu('View') helpMenu = mainMenu.addMenu('Help') printAction = QAction(QIcon("print.png"), "Print", self) printAction.triggered.connect(self.printDialog) fileMenu.addAction(printAction) printPreviewAction = QAction(QIcon("printprev.png"), "Print Preview", self) printPreviewAction.triggered.connect(self.printpreviewDialog) fileMenu.addAction(printPreviewAction) exportpdfAction = QAction(QIcon("pdf.png"), "Export PDF", self) exportpdfAction.triggered.connect(self.printPDF) fileMenu.addAction(exportpdfAction) exiteAction = QAction(QIcon("exit.png"), 'Exit', self) exiteAction.setShortcut("Ctrl+E") exiteAction.triggered.connect(self.exitWindow) fileMenu.addAction(exiteAction) copyAction = QAction(QIcon("copy.png"), 'Copy', self) copyAction.setShortcut("Ctrl+C") editMenu.addAction(copyAction) saveAction = QAction(QIcon("Save.png"), 'Save', self) saveAction.setShortcut("Ctrl+S") editMenu.addAction(saveAction) pasteAction = QAction(QIcon("Paste.png"), 'Paste', self) pasteAction.setShortcut("Ctrl+P") editMenu.addAction(pasteAction) fontAction = QAction(QIcon("font.png"), "Font", self) fontAction.setShortcut("Ctrl+F") fontAction.triggered.connect(self.fontDialog) viewMenu.addAction(fontAction) colorAction = QAction(QIcon("color.png"), "Color", self) colorAction.triggered.connect(self.colorDialog) viewMenu.addAction(colorAction) self.toolbar = self.addToolBar('Toolbar') self.toolbar.addAction(copyAction) self.toolbar.addAction(saveAction) self.toolbar.addAction(pasteAction) self.toolbar.addAction(exiteAction) self.toolbar.addAction(fontAction) self.toolbar.addAction(colorAction) self.toolbar.addAction(printAction) self.toolbar.addAction(printPreviewAction) self.toolbar.addAction(exportpdfAction) def exitWindow(self): self.close() def createEditor(self): self.textEdit = QTextEdit(self) self.setCentralWidget(self.textEdit) def fontDialog(self): font, ok = QFontDialog.getFont() if ok: self.textEdit.setFont(font) def colorDialog(self): color = QColorDialog.getColor() self.textEdit.setTextColor(color) def printDialog(self): printer = QPrinter(QPrinter.HighResolution) dialog = QPrintDialog(printer, self) if dialog.exec_() == QPrintDialog.Accepted: self.textEdit.print_(printer) def printpreviewDialog(self): printer = QPrinter(QPrinter.HighResolution) previewDialog = QPrintPreviewDialog(printer, self) previewDialog.paintRequested.connect(self.printPreview) previewDialog.exec_() def printPreview(self, printer): self.textEdit.print_(printer) |
Now we create a method for printPDF() and we create our PrintPDF code in that method.
1 2 3 4 5 6 7 8 |
def printPDF(self): fn, _ = QFileDialog.getSaveFileName(self, 'Export PDF', None, 'PDF files (.pdf);;All Files()') if fn != '': if QFileInfo(fn).suffix() == "" : fn += '.pdf' printer = QPrinter(QPrinter.HighResolution) printer.setOutputFormat(QPrinter.PdfFormat) printer.setOutputFileName(fn) self.textEdit.document().print_(printer) |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec()) |
Run the complete code this will be the result
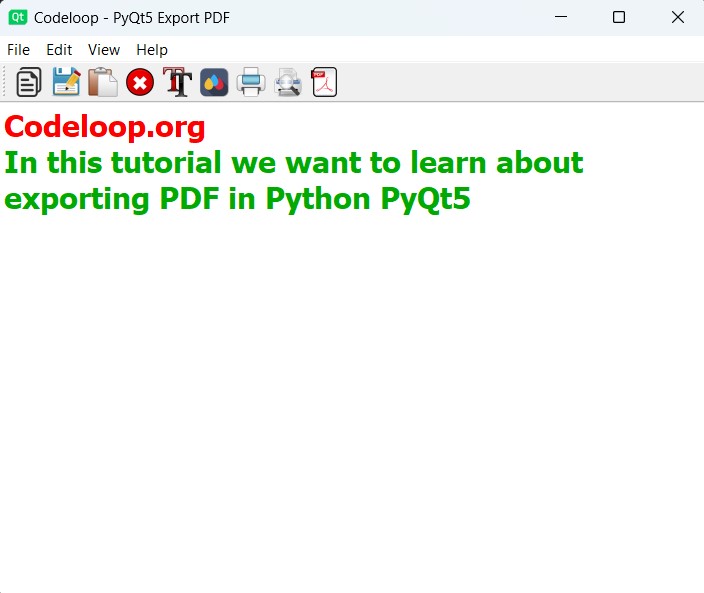
Complete Source Code For This Article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QMainWindow, QAction, QTextEdit, QFontDialog, QColorDialog, QFileDialog import sys from PyQt5.QtGui import QIcon from PyQt5.QtPrintSupport import QPrintDialog, QPrinter, QPrintPreviewDialog from PyQt5.Qt import QFileInfo class Window(QMainWindow): def __init__(self): super().__init__() # Window properties self.title = "Codeloop - PyQt5 Export PDF" self.top = 200 self.left = 500 self.width = 680 self.height = 480 # Set window icon and title self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) # Create text editor self.createEditor() # Create menu bar self.CreateMenu() # Display the window self.show() def CreateMenu(self): # Create menu bar and menus mainMenu = self.menuBar() fileMenu = mainMenu.addMenu('File') editMenu = mainMenu.addMenu('Edit') viewMenu = mainMenu.addMenu('View') helpMenu = mainMenu.addMenu('Help') # Define actions and connect signals printAction = QAction(QIcon("print.png"), "Print", self) printAction.triggered.connect(self.printDialog) fileMenu.addAction(printAction) printPreviewAction = QAction(QIcon("printprev.png"), "Print Preview", self) printPreviewAction.triggered.connect(self.printpreviewDialog) fileMenu.addAction(printPreviewAction) exportpdfAction = QAction(QIcon("pdf.png"), "Export PDF", self) exportpdfAction.triggered.connect(self.printPDF) fileMenu.addAction(exportpdfAction) exitAction = QAction(QIcon("exit.png"), 'Exit', self) exitAction.setShortcut("Ctrl+E") exitAction.triggered.connect(self.exitWindow) fileMenu.addAction(exitAction) copyAction = QAction(QIcon("copy.png"), 'Copy', self) copyAction.setShortcut("Ctrl+C") editMenu.addAction(copyAction) saveAction = QAction(QIcon("Save.png"), 'Save', self) saveAction.setShortcut("Ctrl+S") editMenu.addAction(saveAction) pasteAction = QAction(QIcon("Paste.png"), 'Paste', self) pasteAction.setShortcut("Ctrl+P") editMenu.addAction(pasteAction) fontAction = QAction(QIcon("font.png"), "Font", self) fontAction.setShortcut("Ctrl+F") fontAction.triggered.connect(self.fontDialog) viewMenu.addAction(fontAction) colorAction = QAction(QIcon("color.png"), "Color", self) colorAction.triggered.connect(self.colorDialog) viewMenu.addAction(colorAction) # Create toolbar and add actions self.toolbar = self.addToolBar('Toolbar') self.toolbar.addAction(copyAction) self.toolbar.addAction(saveAction) self.toolbar.addAction(pasteAction) self.toolbar.addAction(exitAction) self.toolbar.addAction(fontAction) self.toolbar.addAction(colorAction) self.toolbar.addAction(printAction) self.toolbar.addAction(printPreviewAction) self.toolbar.addAction(exportpdfAction) def exitWindow(self): # Close the application self.close() def createEditor(self): # Create a QTextEdit widget as central widget self.textEdit = QTextEdit(self) self.setCentralWidget(self.textEdit) def fontDialog(self): # Open font dialog and set font for text editor font, ok = QFontDialog.getFont() if ok: self.textEdit.setFont(font) def colorDialog(self): # Open color dialog and set text color for text editor color = QColorDialog.getColor() self.textEdit.setTextColor(color) def printDialog(self): # Open print dialog and print text editor content printer = QPrinter(QPrinter.HighResolution) dialog = QPrintDialog(printer, self) if dialog.exec_() == QPrintDialog.Accepted: self.textEdit.print_(printer) def printpreviewDialog(self): # Open print preview dialog and show text editor content printer = QPrinter(QPrinter.HighResolution) previewDialog = QPrintPreviewDialog(printer, self) previewDialog.paintRequested.connect(self.printPreview) previewDialog.exec_() def printPreview(self, printer): # Print preview of text editor content self.textEdit.print_(printer) def printPDF(self): # Export text editor content as PDF fn, _ = QFileDialog.getSaveFileName(self, 'Export PDF', None, 'PDF files (.pdf);;All Files()') if fn != '': if QFileInfo(fn).suffix() == "": fn += '.pdf' printer = QPrinter(QPrinter.HighResolution) printer.setOutputFormat(QPrinter.PdfFormat) printer.setOutputFileName(fn) self.textEdit.document().print_(printer) # Create QApplication instance and run the application App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
The above example is a PyQt5 application, and it demonstrates how to export files as PDF. It creates a graphical user interface (GUI) with a text editor where users can enter and edit text. The application includes a menu bar and toolbar with options for printing, previewing and exporting the document as a PDF file.
The main features of the application include:
- We created QMainWindow subclass to define the main window of the application.
- We add actions to the menu bar and toolbar for common operations such as copying, saving, pasting, changing font and color, printing, previewing and exporting as PDF.
- Also we implements dialog windows for font selection, color selection, printing, and print preview.
- And also we use QPrinter to handle printing and PDF export functionalities. The printDialog() function opens a dialog for printing the document, while the printPDF() function allows users to export the document as a PDF file.
FAQs:
What is PyQt5 QPrinter?
PyQt5 QPrinter is a class in the PyQt5 library, and it is used for creating and managing printer objects. These objects can be used to print documents or generate PDF files. QPrinter provides different options such as setting the output format, page layout, number of copies, etc.
Can I export any type of document as PDF in PyQt5?
Yes, you can export different types of documents such as text files, rich text documents, HTML files, etc., as PDF in PyQt5. You can load the document content into a QTextEdit or QTextDocument widget and then use QPrinter to export it as PDF.
Subscribe and Get Free Video Courses & Articles in your Email