In this Python article i want to show you How to Download YouTube Videos with Python, so for this purpose we are going to use third library that is called Pytube3, it is a lightweight, dependency-free Python library (and command-line utility) for downloading YouTube Videos, It has no third party dependencies and aims to be highly reliable. pytube also makes pipelining easy, allowing you to specify callback functions for different download events, such as on progress or on complete.
Learn More
Installation
First of all you need to install this library, make sure that you install pytube3 not pytube, you can install using pip.
1 |
pip install pytube3 |
How to Download YouTube Videos with Python
OK now let’s start our coding.
1 2 3 4 5 6 7 8 |
from pytube import YouTube yt_video = YouTube('https://youtu.be/ym830uzZbQY') print(yt_video.title) print(yt_video.thumbnail_url) print(yt_video.video_id) print(yt_video.views) |
In the above code first we have imported our pytube library, and after that you need to add your video url, now we want to print some information’s about the video like title of the video, thumbnail url, video id and video views.
Run the code and this is the result.
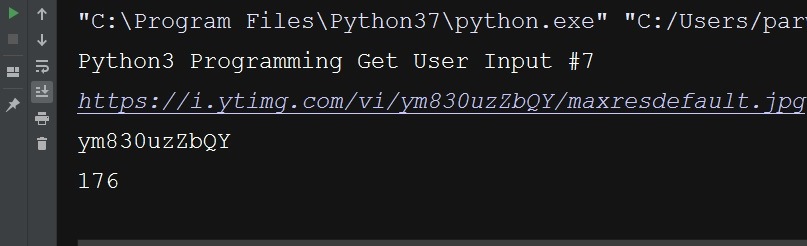
Using this code you can download the video author and video description.
1 2 3 4 5 6 |
from pytube import YouTube yt_video = YouTube('https://youtu.be/ym830uzZbQY') print(yt_video.author) print(yt_video.description) |
Run the code and this is the result.
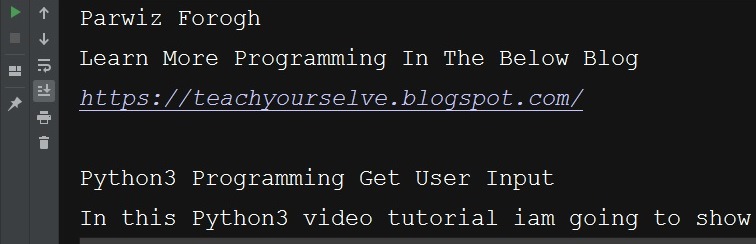
If you want to get available video formats, than you can use this code.
1 2 3 4 5 |
from pytube import YouTube yt_video = YouTube('https://youtu.be/ym830uzZbQY') print(yt_video.streams) |
This is the result.
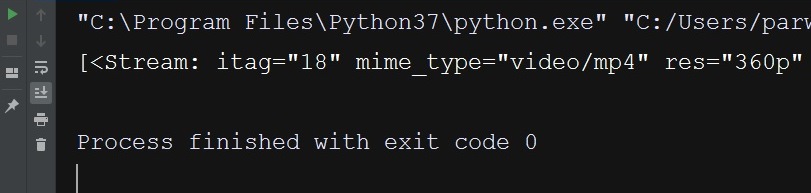
Now we want to Download YouTube Videos, you can simply use download() method for dowloading.
1 2 3 4 5 6 |
from pytube import YouTube yt_video = YouTube('https://youtu.be/ym830uzZbQY') stream = yt_video.streams.first() stream.download() |
Now we want to Download YouTube Playlist
1 2 3 4 |
from pytube import Playlist pl = Playlist("add playlist") pl.download_all |
By using this code you can download the highest resolution video.
1 2 3 4 |
from pytube import YouTube yt_videos = YouTube('https://youtu.be/ym830uzZbQY') yt_videos.streams.get_highest_resolution().download() |
FAQs:
Q: How do I download YouTube videos to MP3 using Python?
A: For downloading YouTube videos as MP3 files using Python, you can use libraries like pytube or youtube_dl to download the video in the desired format (usually as an audio stream), and after that convert it to MP3 using a library like moviepy or ffmpeg. Also you can use third-party services or APIs that offer direct conversion of YouTube videos to MP3.
Q: Is any video downloader using Python?
A: Yes, there are several video downloader libraries and tools available in Python. Some popular ones include pytube, youtube_dl and pafy. These libraries provide APIs for accessing and downloading videos from YouTube and other video sharing platforms.
Q: What is the download code for YouTube videos?
A: The download code for YouTube videos will be different depending on the library or tool you are using. However, in general, you need to provide the URL of the YouTube video you want to download and specify any desired options such as video quality, format, or download location. i already have created the example using Pytube.
Q: How to download a whole playlist from YouTube using Python?
A: To download a whole playlist from YouTube using Python, you can use libraries like pytube, youtube_dl or pafy. These libraries provide methods for fetching all the videos in a playlist and downloading them one by one.
Subscribe and Get Free Video Courses & Articles in your Email