In this OpenCV article iam going to show you How to Detect Corner in OpenCV with CornerHarris. basically in this article we are using CornerHarris algorithm.
Also you can check More GUI Development Tutorials in the below link.
1: PyQt5 GUI Development Tutorials
2: TKinter GUI Development Tutorials
3: Pyside2 GUI Development Tutorials
4: Kivy GUI Development Tutorials
So now this is the complete code for How to Detect Corner in OpenCV with CornerHarris
1 2 3 4 5 6 7 8 9 10 11 |
import cv2 import numpy as np img = cv2.imread('chess_board.png') gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) gray = np.float32(gray) dst = cv2.cornerHarris(gray, 2, 3, 0.04) img[dst>0.01 * dst.max()] = [0, 0, 255] cv2.imshow("HarrisCorner Detection", img) cv2.waitKey() cv2.destroyAllWindows() |
In this article iam using this image
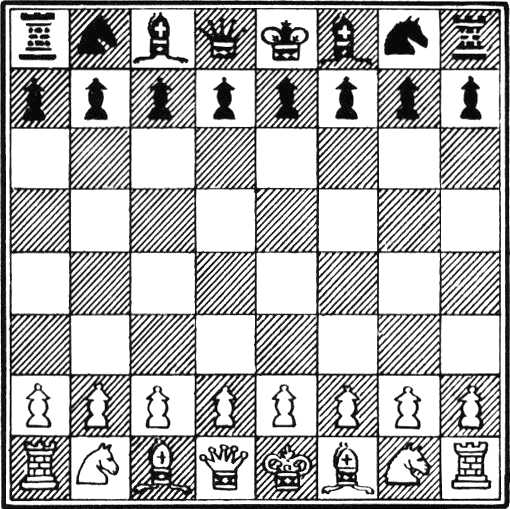
OK in this code first of all we have loaded the image and also we have done conversion on our image, make sure that you have added the image in your working directory.
1 2 3 |
img = cv2.imread('chess_board.png') gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) gray = np.float32(gray) |
So this is the important point and our CornerHarris algorithm
OpenCV has the function cv2.cornerHarris() for this purpose. Its arguments are :
- img – Input image, it should be grayscale and float32 type.
- blockSize – It is the size of neighbourhood considered for corner detection
- ksize – Aperture parameter of Sobel derivative used.
- k – Harris detector free parameter in the equation.
1 |
dst = cv2.cornerHarris(gray, 2, 3, 0.04) |
So now run the complete code and this will be the result
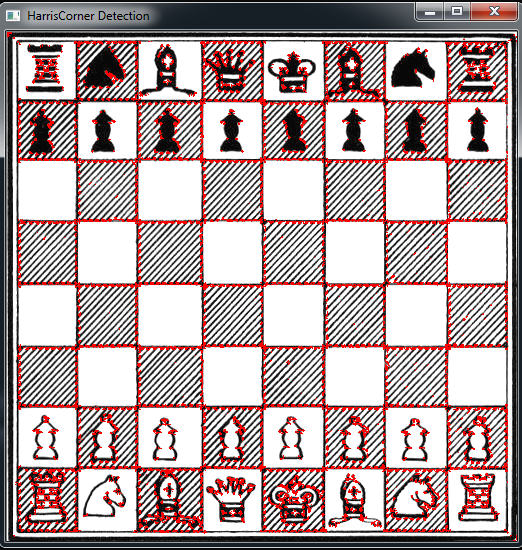
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email