In this PyQt5 article iam going to talk about QtQuick and QML. and also iam going to show you How To Create Window in PyQt5 QtQuick. So first of all what is QtQuick ?
What is QtQuick?
QtQuick is a feature of PyQt5 by using that you can separate your main design from logic. and QtQuick has its own language that is called QML. QML is Qt Markup Language, it is a language similar to JavaScript but it is not JavaScript. So when you want to work with QtQuick you need to create two files in Pycharm IDE, the first one is a QML file and the second one is a Python file.
QtQuick module in PyQt5 includes classes and components for integrating QML with Python applications. Some key features and components of QtQuick in PyQt5 are:
- QQmlApplicationEngine: This class provides an environment for loading and running QML files inside PyQt5 application. It allows you to create Qt applications with QML-based user interfaces.
- QQmlComponent: This class represents a QML component that can be instantiated dynamically at runtime. It enables the creation of QML objects programmatically from Python code.
- QQmlContext: This class manages the context properties and object ownership for QML components. It allows Python objects to be exposed to QML and vice versa, and it enables communication between Python and QML components.
- QQuickView: This class provides a window for displaying QML content. It is similar to QWidget in PyQt, but specifically designed for embedding QML content within PyQt applications.
- QQuickItem: This class represents a visual item in a QML scene. It serves as the base class for all QML items and provides properties and methods for interacting with QML objects from Python.
- QML Elements: QtQuick module includes various QML elements (e.g., Rectangle, Text, Image, ListView) for creating user interfaces. These elements can be customized and styled using QML syntax.
How to Create Window in PyQt5 QtQuick?
Now let’s create our example, this is our QML file for How to Create Window in PyQt5 QtQuick.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import QtQuick.Window 2.2 import QtQuick 2.3 Window { visible:true width:600 height:400 color:"yellow" title: "Codeloop - PyQt5 QML Window" } |
This code creates a window with a yellow background and a title. It’s visible and has a size of 600 pixels wide and 400 pixels tall.
Now it is time to load our this QML file in Python. write the below code for loading.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
# Import necessary modules from PyQt5.QtQml import QQmlApplicationEngine from PyQt5.QtWidgets import QApplication from PyQt5.QtGui import QIcon import sys # Function to run QML application def runQML(): # Create QApplication instance app = QApplication(sys.argv) # Create QQmlApplicationEngine instance to load and run QML files engine = QQmlApplicationEngine() # Set the application icon app.setWindowIcon(QIcon("codeloop.png")) # Load main QML file engine.load('main.qml') # Check if loading the QML file was successful if not engine.rootObjects(): return -1 # Execute the application and return the exit code return app.exec_() # Main entry point of the script if __name__ == "__main__": # Run the QML application and exit with the return code sys.exit(runQML()) |
At the top these are the imports.
1 2 3 4 |
from PyQt5.QtQml import QQmlApplicationEngine from PyQt5.QtWidgets import QApplication from PyQt5.QtGui import QIcon import sys |
This is the place that we want to load our qml file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
def runQML(): app =QApplication(sys.argv) engine = QQmlApplicationEngine() app.setWindowIcon(QIcon("icon.png")) engine.load('main.qml') if not engine.rootObjects(): return -1 return app.exec_() if __name__ == "__main__": sys.exit(runQML()) |
So run the code and this will be the result.
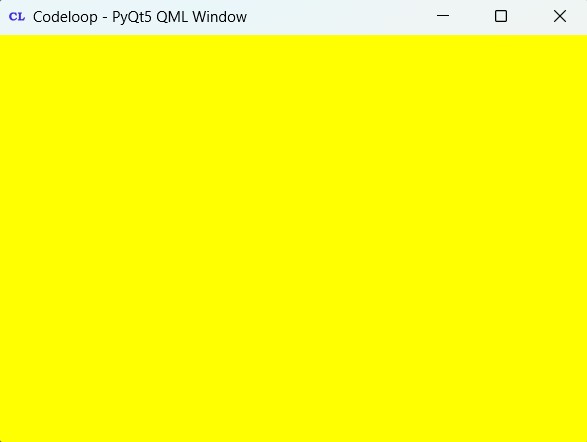
Subscribe and Get Free Video Courses & Articles in your Email