In this wxPython article iam going to show How to Create Tooltip in wxPython. first of all let’s talk about wxPython Tooltip.
What is wxPython Tooltip?
A wxPython tooltip is a small informational window that appears when the user hovers the mouse cursor over a specific widget or area of a graphical user interface (GUI) built with wxPython. Tooltips provide additional context or explanation about the purpose or function of a particular GUI element, such as a button, text box or image.
In wxPython, tooltips can be easily added to widgets using the wx.ToolTip class. You can customize the content, appearance, and behavior of tooltips, including their position, delay before appearing and duration of display.
How to Create Tooltip in wxPython?
Now let’s create our example, so now this is the complete code for How To Create Tooltip in wxPython.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import wx class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title, size=(300, 200)) # Set window icon self.SetIcon(wx.Icon("codeloop.png")) self.panel = MyPanel(self) class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) # Create tooltips self.tipClick = wx.ToolTip('This Is Click Tooltip') self.tipOpen = wx.ToolTip('This Is Open Tooltip') # Create buttons self.button = wx.Button(self, label="Click", pos=(80, 80)) self.button.SetToolTip(self.tipClick) self.button2 = wx.Button(self, label="Open", pos=(80, 130)) self.button2.SetToolTip(self.tipOpen) class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Codeloop.org - wxPython Tooltip") self.frame.Show() return True app = MyApp() app.MainLoop() |
So this class is MyFrame class that inherits from wx.Frame and it is a top level window that contains our panel.
1 2 3 4 5 6 7 8 |
class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title =title) self.panel = MyPanel(self) |
After that we have MyPanel class that inherits from wx.Panel and it is the place that we create our widgets and layouts in this class. also we are going to create our two buttons with tooltip in this class.
1 2 3 4 5 6 7 8 9 10 11 |
class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) self.tipClick = wx.ToolTip('This Is Click Tooltip') self.tipOpen =wx.ToolTip('This Is Open Tooltip') self.button = wx.Button(self, label = "Click", pos = (80,80)) self.button.SetToolTip(self.tipClick) self.button2 = wx.Button(self, label = "Open", pos = (80,130)) self.button2.SetToolTip(self.tipOpen) |
Basically we are going to create two buttons with two Tooltip , so this is for creating of Tooltip and buttons. also we set the Tooltip for our buttons.
1 2 3 4 5 6 7 |
self.tipClick = wx.ToolTip('This Is Click Tooltip') self.tipOpen =wx.ToolTip('This Is Open Tooltip') self.button = wx.Button(self, label = "Click", pos = (80,80)) self.button.SetToolTip(self.tipClick) self.button2 = wx.Button(self, label = "Open", pos = (80,130)) self.button2.SetToolTip(self.tipOpen) |
So the last class is MyApp class that inherits from wx.App. the OnInit() method is where you will most often create frame subclass objects. and start our main loop.That’s it. Once the application’s main event loop processing takes over, control passes to wxPython. Unlike procedural programs, a wxPython GUI program primarily responds to the events taking place around it, mostly determined by a human user clicking with a mouse and typing at the keyboard. When all the frames in an application have been closed, the app.MainLoop() method will return and the program will exit.
1 2 3 4 5 6 7 8 9 10 |
class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Codeloop.org - wxPython Tooltip") self.frame.Show() return True app = MyApp() app.MainLoop() |
Run the code and this will be the result
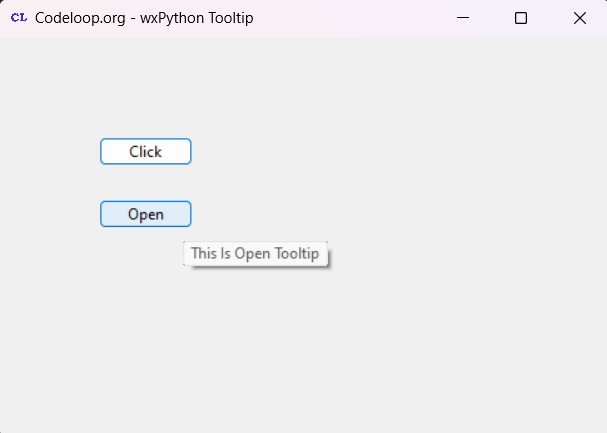
Subscribe and Get Free Video Courses & Articles in your Email