In this wxPython article i want to show you How to Create TextControl in wxPython. so a text control allows text to be displayed and edited. It may be single line or multi-line. Notice that a lot of methods of the text controls are found in the base wx.TextEntry class which is a common base class for wx.TextCtrl and other controls using a single line text entry field (e.g. wx.ComboBox).
wxPython Window Styles
This class supports the following styles:
- wx.TE_PROCESS_ENTER: The control will generate the event wxEVT_TEXT_ENTER (otherwise pressing Enter key is either processed internally by the control or used to activate the default button of the dialog, if any).
- wx.TE_PROCESS_TAB: Normally, TAB key is used for keyboard navigation and pressing it in a control switches focus to the next one. With this style, this won’t happen and if the TAB is not otherwise processed (e.g. by wxEVT_CHAR event handler), a literal TAB character is inserted into the control. Notice that this style has no effect for single-line text controls when using wxGTK.
- wx.TE_MULTILINE: The text control allows multiple lines. If this style is not specified, line break characters should not be used in the controls value.
- wx.TE_PASSWORD: The text will be echoed as asterisks.
- wx.TE_READONLY: The text will not be user-editable.
- wx.TE_RICH: Use rich text control under MSW, this allows having more than 64KB of text in the control. This style is ignored under other platforms.
- wx.TE_RICH2: Use rich text control version 2.0 or higher under MSW, this style is ignored under other platforms
- wx.TE_AUTO_URL: Highlight the URLs and generate the TextUrlEvents when mouse events occur over them.
- wx.TE_NOHIDESEL: By default, the Windows text control doesn’t show the selection when it doesn’t have focus – use this style to force it to always show it. It doesn’t do anything under other platforms.
- wx.TE_LEFT: The text in the control will be left-justified (default).
- wx.TE_CENTRE: The text in the control will be centered (wxMSW, wxGTK, wxOSX).
How to Create TextControl in wxPython?
Let’s create our example, This is the complete code for How to Create TextControl in wxPython.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
import wx # Define main frame class class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title, size=(800, 600)) # Set window icon self.SetIcon(wx.Icon('codeloop.png')) # Create instance of the panel class panel = MyPanel(self) # Define panel class class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) # Create multiline text control widget self.textCtrl = wx.TextCtrl(self, size=(200, 100), style=wx.TE_MULTILINE) # Define application class class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Codeloop.org - wxPython TextControl") self.frame.Show() return True # Create an instance of the application app = MyApp() # Start the application event loop app.MainLoop() |
Now let’s describe the code:
- Imports: This code imports wx module, and it provides wxPython library for creating GUI applications.
- MyFrame class: This class represents the main application window. It inherits from wx.Frame. In the constructor (__init__), it sets the window title, size and icon using SetIcon.
- MyPanel class: This class represents a panel inside the main window where UI components are placed. It inherits from wx.Panel. In the constructor, it creates a multiline text control widget (wx.TextCtrl) and adds it to the panel.
- MyApp class: This class initializes and starts the wxPython application. It inherits from wx.App. In the OnInit method, it creates an instance of MyFrame, sets it as the main application window, and displays it.
- Application Execution: An instance of MyApp is created, and the MainLoop method is called to start the event loop, it allows the application to respond to user input and events.
So run the code and this will be the result
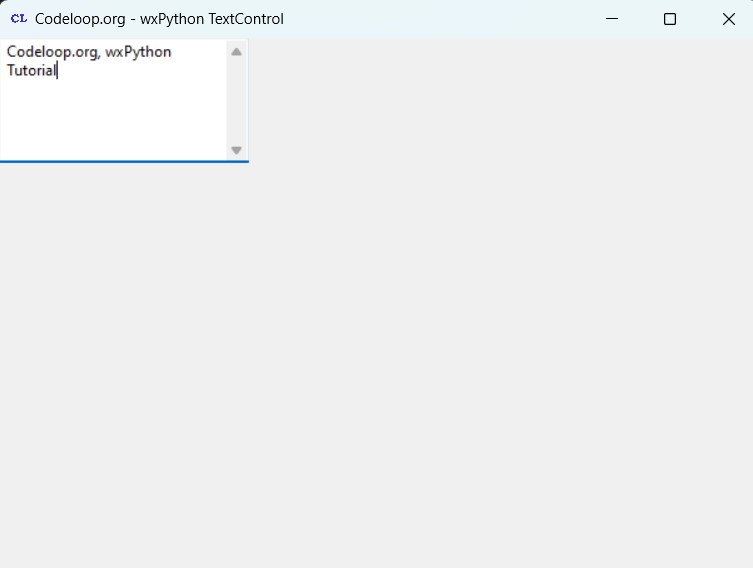
Subscribe and Get Free Video Courses & Articles in your Email