In this wxPython article i want to show How to Create SpinControl in wxPython. wx.SpinCtrl combines wx.TextCtrl and wx.SpinButton in one control.
So This class supports the following styles:
- wx.SP_ARROW_KEYS: The user can use arrow keys to change the value.
- wx.SP_WRAP: The value wraps at the minimum and maximum.
- wx.TE_PROCESS_ENTER: Indicates that the control should generate wxEVT_TEXT_ENTER events. Using this style will prevent the user from using the Enter key for dialog navigation (e.g. activating the default button in the dialog) under MSW.
wx.ALIGN_LEFT: Same as wx.TE_LEFT for wx.TextCtrl: the text is left aligned (this is the default).
How to Create SpinControl in wxPython?
Now let’s create our example, This is the complete code for How to Create SpinControl in wxPython
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
import wx class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title, size=(400, 200)) self.panel = MyPanel(self) class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) self.spinControl = wx.SpinCtrl(self, -1, "", (30, 50)) self.spinControl.SetRange(1, 100) self.spinControl.SetValue(5) self.label = wx.StaticText(self, label="", pos=(30, 80)) self.Bind(wx.EVT_SPINCTRL, self.OnSpin, self.spinControl) def OnSpin(self, evt): spinValue = self.spinControl.GetValue() self.label.SetLabelText("The Value Is: " + str(spinValue)) class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Codeloop.org - wxPython SpinControl") self.frame.SetIcon(wx.Icon("codeloop.png", wx.BITMAP_TYPE_PNG)) self.frame.Show() return True app = MyApp() app.MainLoop() |
So this class is MyFrame class that inherits from wx.Frame and it is a top level window that contains our panel.
1 2 3 4 5 6 7 8 9 |
class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title =title) self.panel = MyPanel(self) |
After that we have MyPanel class that inherits from wx.Panel and it is the place that we create our widgets and layouts in this class. in this class we create our SpinControl with a StaticText.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) self.spinControl = wx.SpinCtrl(self, -1, "", (30, 50)) self.spinControl.SetRange(1, 100) self.spinControl.SetValue(5) self.label = wx.StaticText(self, label = "", pos = (30,80)) self.Bind(wx.EVT_SPINCTRL, self.OnSpin, self.spinControl) |
These are for creating SpinControl also we set the range value for our SpinControl
1 2 3 |
self.spinControl = wx.SpinCtrl(self, -1, "", (30, 50)) self.spinControl.SetRange(1, 100) self.spinControl.SetValue(5) |
This is our label or we can call it StaticText
1 |
self.label = wx.StaticText(self, label = "", pos = (30,80)) |
In here we bind a method to our SpinControl
1 |
self.Bind(wx.EVT_SPINCTRL, self.OnSpin, self.spinControl) |
This is the event method for our SpinControl
1 2 3 |
def OnSpin(self, evt): spinValue = self.spinControl.GetValue() self.label.SetLabelText("The Value Is : " + str(spinValue)) |
So the last class is MyApp class that inherits from wx.App. the OnInit() method is where you will most often create frame subclass objects. and start our main loop.That’s it. Once the application’s main event loop processing takes over, control passes to wxPython. Unlike procedural programs, a wxPython GUI program primarily responds to the events taking place around it, mostly determined by a human user clicking with a mouse and typing at the keyboard. When all the frames in an application have been closed, the app.MainLoop() method will return and the program will exit.
1 2 3 4 5 6 7 8 9 10 |
class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="wxPython - Spin Control") self.frame.Show() return True app = MyApp() app.MainLoop() |
So run the code and this will be the result
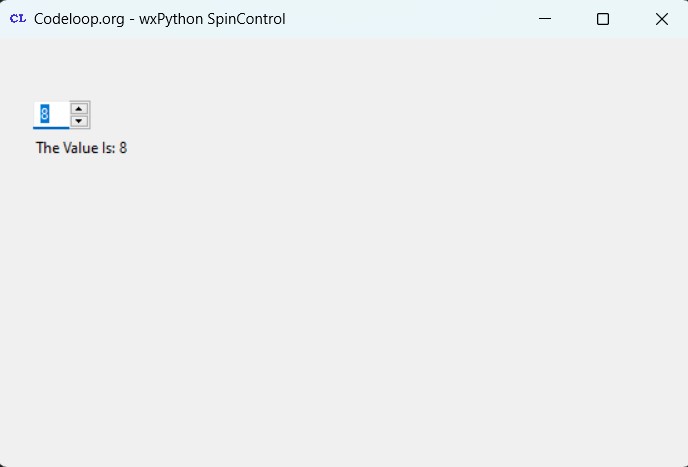
FAQs:
What is the latest version of wxPython?
The latest version of wxPython as of my last update is wxPython 4.2.1. This version includes different enhancements, bug fixes, and new features compared to previous versions.
What is wxPython used for?
wxPython is a GUI library for Python programming language. Using wxPython you can create native cross-platform applications with a graphical user interface. wxPython provides different widgets and tools for building desktop applications, including buttons, text controls, menus and many more.
What platforms are supported by wxPython?
wxPython supports multiple platforms, including Windows, macOS and different Linux distributions. This cross-platform compatibility makes it the best choice for developing desktop applications that can run across different different operating systems.
Subscribe and Get Free Video Courses & Articles in your Email