In this wxPython article i want to show you How to Create Slider in wxPython. So a slider is a control with handle which can be pulled back and forth to change the value. On Windows, the track bar control is used. Slider generates the same events as wx.ScrollBar, but in practice the most convenient way to process wx.Slider updates is by handling the slider specific wxEVT_SLIDER event which carries wx.CommandEvent containing just the latest slider position.
How to Create Slider in wxPython?
Now let’s create our example, so this is the complete code for How to Create Slider in wxPython.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
import wx class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title=title, size=(400, 300)) # Set icon for the frame self.SetIcon(wx.Icon("codeloop.png")) # Initialize panel self.panel = MyPanel(self) class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) # Create vertical box sizer to manage the layout vbox = wx.BoxSizer(wx.VERTICAL) # Create slider with horizontal orientation and labels self.slider = wx.Slider(self, value=10, minValue=1, maxValue=100, style=wx.SL_HORIZONTAL | wx.SL_LABELS) # Add slider to the sizer with expansion and top border vbox.Add(self.slider, 0, flag=wx.EXPAND | wx.TOP, border=20) # Bind slider event to the OnSliderScroll method self.Bind(wx.EVT_SLIDER, self.OnSliderScroll) # Create static text widget to display a label self.txt = wx.StaticText(self, label='Codeloop', style=wx.ALIGN_CENTER) # Add static text to the sizer vbox.Add(self.txt, 0, flag=wx.ALIGN_CENTER_HORIZONTAL | wx.TOP, border=20) # Set the sizer for the panel self.SetSizer(vbox) # Center the frame on the screen self.Center() def OnSliderScroll(self, event): # Get the slider value obj = event.GetEventObject() value = obj.GetValue() # Change the font size of the static text font = self.GetFont() font.SetPointSize(value) self.txt.SetFont(font) class MyApp(wx.App): def OnInit(self): # Initialize main frame with a title and show it self.frame = MyFrame(parent=None, title="Codeloop.org - WxPython Slider") self.frame.Show() return True # Entry point of the application app = MyApp() app.MainLoop() |
At the first we have our Frame class that inherits from wx.Frame and it is our top level window that we create our MyPanel object in this class.
1 2 3 4 5 6 |
class MyFrame(wx.Frame): def __init__(self, parent, title): super(MyFrame, self).__init__(parent, title =title, size = (400,300)) self.panel = MyPanel(self) |
After that we create our MyPanel class, this class is a container class for our widgets like buttons, menus, checkbutton and etc. you can see that we have created our Slider in this class.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
class MyPanel(wx.Panel): def __init__(self, parent): super(MyPanel, self).__init__(parent) vbox = wx.BoxSizer(wx.VERTICAL) self.slider = wx.Slider(self, value=10, minValue=1, maxValue=100, style=wx.SL_HORIZONTAL | wx.SL_LABELS) vbox.Add(self.slider,1, flag = wx.EXPAND | wx.ALIGN_CENTER_HORIZONTAL | wx.TOP, border = 20) self.Bind(wx.EVT_SLIDER, self.OnSliderScroll) self.txt = wx.StaticText(self, label='Hello', style=wx.ALIGN_CENTER) vbox.Add(self.txt, 1, wx.ALIGN_CENTRE_HORIZONTAL) self.SetSizer(vbox) self.Center() |
This is for creation of our vertical box sizer
1 |
vbox = wx.BoxSizer(wx.VERTICAL) |
So in here we have created our slider and we have added our slider to the vertical box sizer.
1 2 3 4 |
self.slider = wx.Slider(self, value=10, minValue=1, maxValue=100, style=wx.SL_HORIZONTAL | wx.SL_LABELS) vbox.Add(self.slider,1, flag = wx.EXPAND | wx.ALIGN_CENTER_HORIZONTAL | wx.TOP, border = 20) |
And in here we want to bind our method to Slider
1 |
self.Bind(wx.EVT_SLIDER, self.OnSliderScroll) |
This is a text that we need for our example
1 2 |
self.txt = wx.StaticText(self, label='Hello', style=wx.ALIGN_CENTER) vbox.Add(self.txt, 1, wx.ALIGN_CENTRE_HORIZONTAL) |
So this is the event method that we have bonded in the above to our slider. this method for text, i want when the user change the slider i want the text became bigger
1 2 3 4 5 6 |
def OnSliderScroll(self, event): obj = event.GetEventObject() value = obj.GetValue() font = self.GetFont() font.SetPointSize(self.slider.GetValue()) self.txt.SetFont(font) |
So the last class is MyApp class that inherits from wx.App. the OnInit() method is where you will most often create frame subclass objects. and start our main loop.That’s it. Once the application’s main event loop processing takes over, control passes to wxPython. Unlike procedural programs, a wxPython GUI program primarily responds to the events taking place around it, mostly determined by a human user clicking with a mouse and typing at the keyboard. When all the frames in an application have been closed, the app.MainLoop() method will return and the program will exit.
1 2 3 4 5 6 7 8 9 10 |
class MyApp(wx.App): def OnInit(self): self.frame = MyFrame(parent=None, title="Wxpython Slider") self.frame.Show() return True app = MyApp() app.MainLoop() |
Run the complete code and this will be the result
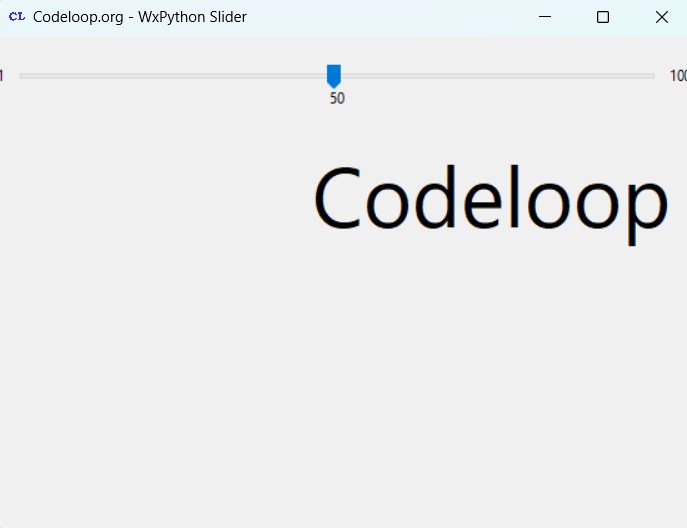
Subscribe and Get Free Video Courses & Articles in your Email