In this TKinter Python article i want to show you How to Create ScrollTextArea in TKinter Python, but first of all let’s talk about ScrollTextArea in TKinter.
What is ScrollTextArea in TKinter?
ScrollTextArea is not a standard widget in Tkinter. However, Tkinter provides a Text widget that can be used to create a text area with scrolling capabilities. This widget is suitable for displaying and editing multiline text. By default, the Text widget doesn’t have a scrollbar, but you can add one by using the Scrollbar widget and configuring it to scroll the Text widget.
How to Create ScrollTextArea in TKinter Python?
For creating scrollable text area in Tkinter, you typically follow these steps:
- Create a Text widget to display the multiline text.
- Create a Scrollbar widget and attach it to the Text widget.
- Configure the Scrollbar to control the scrolling of the Text widget.
- Pack or grid both the Text widget and the Scrollbar widget in the desired layout.
This is the complete code for creating ScrollTextArea in tkinter
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
import tkinter as tk from tkinter import scrolledtext # Create custom Window class inheriting from tk.Tk class Window(tk.Tk): def __init__(self): # Initialize the parent class (tk.Tk) tk.Tk.__init__(self) # Set the title of the window self.title("Codeloop.org - Tkinter ScrollBar") # Set window icon self.iconphoto(True, tk.PhotoImage(file='codeloop.png')) # Call the method to create scrolled text control self.scrolledTextCtrl() # Method to create scrolled text control def scrolledTextCtrl(self): # Define the width and height scroll_w = 30 scroll_h = 10 # Create a ScrolledText widget scrollText = scrolledtext.ScrolledText(self, width=scroll_w, height=scroll_h, wrap=tk.WORD) # Place the ScrolledText widget in the grid layout scrollText.grid(column=0, columnspan=3) # Create an instance of the Window class window = Window() window.mainloop() |
This Python code is a Tkinter application that generates a window with a scrolled text area. It begins by importing necessary modules: tkinter for GUI components and scrolledtext for the scrolled text widget. Also we have defined a custom class Window, it inherits from tk.Tk, representing the main window. In the __init__ method of Window, we have window’s title and icon, also the scrolledTextCtrl method is called to create the scrolled text control. scrolledTextCtrl method establishes the dimensions of the scrolled text area, creates a ScrolledText widget, and places it in the grid layout. And lastly an instance of Window is created, and the Tkinter event loop is initiated.
Run the complete source code and this will be the result.
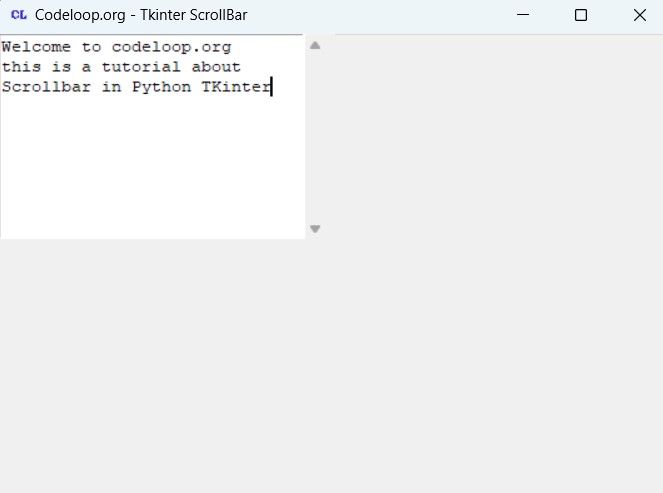
FAQs:
How to create scrollable text in Tkinter?
For creating scrollable text in Tkinter, you can use scrolledtext module. First, import tkinter and scrolledtext. After that create a ScrolledText widget and specify its dimensions and wrapping mode. And lastly add text to the widget using the insert method.
This is an example:
1 2 3 4 5 6 7 8 |
import tkinter as tk from tkinter import scrolledtext root = tk.Tk() text_area = scrolledtext.ScrolledText(root, width=30, height=10, wrap=tk.WORD) text_area.insert(tk.END, "Your text here") text_area.pack() root.mainloop() |
How do I add a scroll to Tkinter?
For adding a scrollbar to Tkinter, you can use the Scrollbar widget along with a widget that supports scrolling, such as Canvas, Listbox or Text. After that link the scrollbar to the widget using the yscrollcommand parameter.
This is an example using a Listbox widget:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import tkinter as tk root = tk.Tk() scrollbar = tk.Scrollbar(root) listbox = tk.Listbox(root, yscrollcommand=scrollbar.set) scrollbar.config(command=listbox.yview) # Add items to the listbox for i in range(50): listbox.insert(tk.END, f"Item {i}") scrollbar.pack(side=tk.RIGHT, fill=tk.Y) listbox.pack(side=tk.LEFT, fill=tk.BOTH, expand=True) root.mainloop() |
How do I make a textbox scrollable?
For making a textbox scrollable in Tkinter, you can use ScrolledText widget from the scrolledtext module. This widget provides built-in scrolling functionality for text areas.
This is an example:
1 2 3 4 5 6 7 |
from tkinter import scrolledtext import tkinter as tk root = tk.Tk() text_area = scrolledtext.ScrolledText(root, width=30, height=10, wrap=tk.WORD) text_area.pack() root.mainloop() |
Subscribe and Get Free Video Courses & Articles in your Email