In this article i want to show you How To Create QTabWidget in PyQt5, we are using QTabWidget class for this. the QTabWidget class provides a stack of tabbed widgets. a tab widget provides a tab bar and a “page area” that is used to display pages related to each tab. By default, the tab bar is shown above the page area, but different configurations are available
What is QTabWidget in PyQt5 ?
In PyQt5 QTabWidget is a widget that provides tabbed interface for displaying multiple widgets within single window. it allows you to switch between different pages or tabs, each of which can contain its own set of widgets. QTabWidget widget is commonly used in graphical user interfaces (GUIs) to display multiple views or modes within a single application.
each tab in QTabWidget is associated with widget that is displayed when the tab is selected. you can use the addTab() method to add a new tab and assign a widget to it. the widget can be any PyQt5 widget, such as QLabel, QPushButton or QComboBox.
QTabWidget provides several methods to customize the appearance and behavior of the tabs, such as setTabText() to set the text of a tab, setTabToolTip() to set the tooltip of a tab, and setTabEnabled() to enable or disable a tab. You can also use the currentChanged signal to detect when the user changes the selected tab.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Pyside2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
First we need some imports
1 2 3 4 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QDialog,QTabWidget, QComboBox, QCheckBox ,QGroupBox ,QVBoxLayout, QWidget, QLabel, QLineEdit, QDialogButtonBox import sys from PyQt5.QtGui import QIcon |
After that we are going to create our main window class that extends from QDialog and we have added our window requirements in the constructor of that class. for example we have set the window title, window icon.
1 2 3 4 5 6 |
class Tab(QDialog): def __init__(self): super().__init__() self.setWindowTitle("PyQt5 TabWidget Example") self.setWindowIcon(QIcon("icon.png")) #self.setStyleSheet('background-color:grey') |
If you want to give a color for your window you can use this code.
1 |
#self.setStyleSheet('background-color:grey') |
So now we need to create the object of QVBoxLayout, QTabWidget and also QDialogButtonBox. the QDialogButtonBox class is a widget that presents buttons in a layout that is appropriate to the current widget style. Dialogs and message boxes typically present buttons in a layout that conforms to the interface guidelines for that platform. Invariably, different platforms have different layouts for their dialogs. QDialogButtonBox allows a developer to add buttons to it and will automatically use the appropriate layout for the user’s desktop environment.
Most buttons for a dialog follow certain roles. Such roles include:
- Accepting or rejecting the dialog.
- Asking for help.
- Performing actions on the dialog itself (such as resetting fields or applying changes).
1 2 3 |
vbox = QVBoxLayout() tabWidget = QTabWidget() buttonbox = QDialogButtonBox(QDialogButtonBox.Ok | QDialogButtonBox.Cancel) |
These are the roles for our button box.
1 2 |
buttonbox.accepted.connect(self.accept) buttonbox.rejected.connect(self.reject) |
In here iam going to set the font for my TabWidget.
1 |
tabWidget.setFont(QtGui.QFont("Sanserif", 12)) |
OK now we are going to add our two tab classes in our TabWidget.
1 2 |
tabWidget.addTab(TabContact(), "Contact Details") tabWidget.addTab(TabPeronsalDetails(), "Personal Details") |
Also we need to add our tabwidget and buttonbox to the vbox layout.
1 2 3 |
vbox.addWidget(tabWidget) vbox.addWidget(buttonbox) self.setLayout(vbox) |
And this is our two tab class that we have already added to our TabWidget. and we have added some widgets in these two tabs.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
class TabContact(QWidget): def __init__(self): super().__init__() nameLabel = QLabel("Name: ") nameEdit = QLineEdit() phone = QLabel("Phone:") phoneedit = QLineEdit() addr = QLabel("Address:") addredit = QLineEdit() email = QLabel("Email:") emailedit = QLineEdit() vbox = QVBoxLayout() vbox.addWidget(nameLabel) vbox.addWidget(nameEdit) vbox.addWidget(phone) vbox.addWidget(phoneedit) vbox.addWidget(addr) vbox.addWidget(addredit) vbox.addWidget(email) vbox.addWidget(emailedit) self.setLayout(vbox) class TabPeronsalDetails(QWidget): def __init__(self): super().__init__() groupBox = QGroupBox("Select Your Gender") list = ["Male", "Female"] combo = QComboBox() combo.addItems(list) vbox = QVBoxLayout() vbox.addWidget(combo) groupBox.setLayout(vbox) groupBox2 = QGroupBox("Select Your Favorite Programming Language") python =QCheckBox("Python") cpp = QCheckBox("C++") java = QCheckBox("Java") csharp = QCheckBox("C#") vboxp = QVBoxLayout() vboxp.addWidget(python) vboxp.addWidget(cpp) vboxp.addWidget(java) vboxp.addWidget(csharp) groupBox2.setLayout(vboxp) mainLayout = QVBoxLayout() mainLayout.addWidget(groupBox) mainLayout.addWidget(groupBox2) self.setLayout(mainLayout) |
Also every PyQt5 application must create an application object.
1 |
app = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 3 |
tabdialog = Tab() tabdialog.show() app.exec_() |
Complete source code for this article
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QDialog,QTabWidget, QComboBox, QCheckBox ,QGroupBox ,QVBoxLayout, QWidget, QLabel, QLineEdit, QDialogButtonBox import sys from PyQt5.QtGui import QIcon class Tab(QDialog): def __init__(self): super().__init__() self.setWindowTitle("PyQt5 TabWidget Example") self.setWindowIcon(QIcon("icon.png")) #self.setStyleSheet('background-color:grey') vbox = QVBoxLayout() tabWidget = QTabWidget() buttonbox = QDialogButtonBox(QDialogButtonBox.Ok | QDialogButtonBox.Cancel) buttonbox.accepted.connect(self.accept) buttonbox.rejected.connect(self.reject) tabWidget.setFont(QtGui.QFont("Sanserif", 12)) tabWidget.addTab(TabContact(), "Contact Details") tabWidget.addTab(TabPeronsalDetails(), "Personal Details") vbox.addWidget(tabWidget) vbox.addWidget(buttonbox) self.setLayout(vbox) class TabContact(QWidget): def __init__(self): super().__init__() nameLabel = QLabel("Name: ") nameEdit = QLineEdit() phone = QLabel("Phone:") phoneedit = QLineEdit() addr = QLabel("Address:") addredit = QLineEdit() email = QLabel("Email:") emailedit = QLineEdit() vbox = QVBoxLayout() vbox.addWidget(nameLabel) vbox.addWidget(nameEdit) vbox.addWidget(phone) vbox.addWidget(phoneedit) vbox.addWidget(addr) vbox.addWidget(addredit) vbox.addWidget(email) vbox.addWidget(emailedit) self.setLayout(vbox) class TabPeronsalDetails(QWidget): def __init__(self): super().__init__() groupBox = QGroupBox("Select Your Gender") list = ["Male", "Female"] combo = QComboBox() combo.addItems(list) vbox = QVBoxLayout() vbox.addWidget(combo) groupBox.setLayout(vbox) groupBox2 = QGroupBox("Select Your Favorite Programming Language") python =QCheckBox("Python") cpp = QCheckBox("C++") java = QCheckBox("Java") csharp = QCheckBox("C#") vboxp = QVBoxLayout() vboxp.addWidget(python) vboxp.addWidget(cpp) vboxp.addWidget(java) vboxp.addWidget(csharp) groupBox2.setLayout(vboxp) mainLayout = QVBoxLayout() mainLayout.addWidget(groupBox) mainLayout.addWidget(groupBox2) self.setLayout(mainLayout) if __name__ == "__main__": app = QApplication(sys.argv) tabdialog = Tab() tabdialog.show() app.exec_() |
Run the code and this is the result
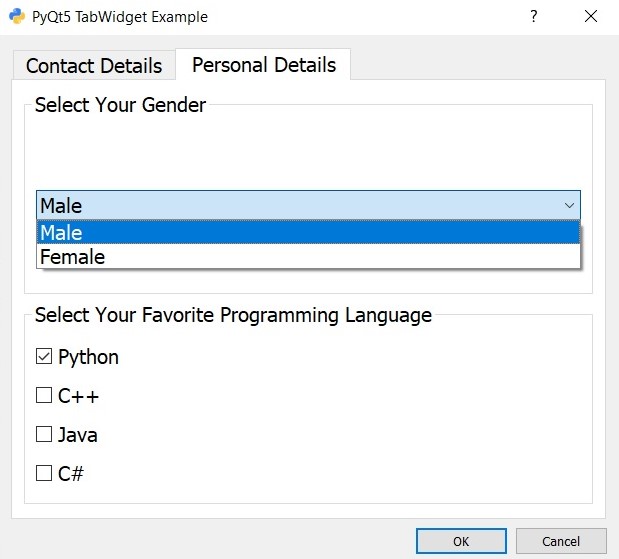
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email