In this PyQt5 article we are going to talk about How To Create QSlider In PyQt5. we are using QSlider class for creating slider in PyQt5 also iam going to show you how you can use valueChanged() signal with PyQt5 Slider. The slider is the classic widget for controlling a bounded value. It lets the user move a slider handle along a horizontal or vertical groove and translates the handle’s position into an integer value within the legal range.
What is PyQt5 QSlider ?
PyQt5 QSlider is graphical user interface (GUI) widget provided by the PyQt5 library. it is horizontal or vertical bar that the user can slide to select a value within specified range. QSlider widget is commonly used as a user interface control for adjusting values such as volume, brightness or the position of video playback.
PyQt5 QSlider widget provides several properties that allow the developer to customize its appearance and behavior. for example minimum and maximum values of the slider can be set, as well as the current value. widget can also be set to either snap to discrete values or to allow for continuous values.
also to its properties PyQt5 QSlider widget provides several signals that can be used to respond to user interaction with the slider. for example valueChanged signal is emitted whenever the user moves the slider to a new value.
we can say that PyQt5 QSlider is useful widget for creating interactive GUI applications with slider interface. it can be customized to match the look and feel of the application, and its signals can be used to update other parts of the GUI or to perform other actions in response to user input.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Pyside2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
First we need some imports.
1 2 3 4 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QWidget, QHBoxLayout, QSlider, QLabel import sys from PyQt5.QtCore import Qt |
After that we are going to create our main Window class that extends from QWidget. and in the constructor of the class we need to add some requirements of the window like set window title, window icon and window geometry.
1 2 3 4 5 6 7 8 9 10 11 |
class Window(QWidget): def __init__(self): super().__init__() self.title = "PyQt5 Slider" self.top = 200 self.left = 500 self.width = 400 self.height = 300 self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) |
In here we have created the object of QHBoxLayout and QSlider, because we are going to use QHBoxLayout.
1 2 |
hbox = QHBoxLayout() self.slider = QSlider() |
We set the orientation for our QSlider and also we have set the the maximum and minimum value for the QSlider.
1 2 3 4 5 |
self.slider.setOrientation(Qt.Horizontal) self.slider.setTickPosition(QSlider.TicksBelow) self.slider.setTickInterval(1) self.slider.setMinimum(0) self.slider.setMaximum(100) |
Now we are going to connect the valueChanged signal of slider with our changedValue method that we will create.
1 |
self.slider.valueChanged.connect(self.changedValue) |
This is our method that we have already connected to QSlider signal at the top. first we have received the value from QSlider and after we have set that value to the QLabel.
1 2 3 |
def changedValue(self): size = self.slider.value() self.label.setText(str(size)) |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec_()) |
Complete source code for How To Create QSlider In PyQt5
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QWidget, QHBoxLayout, QSlider, QLabel import sys from PyQt5.QtCore import Qt class Window(QWidget): def __init__(self): super().__init__() self.title = "PyQt5 Slider" self.top = 200 self.left = 500 self.width = 400 self.height = 300 self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) hbox = QHBoxLayout() self.slider = QSlider() self.slider.setOrientation(Qt.Horizontal) self.slider.setTickPosition(QSlider.TicksBelow) self.slider.setTickInterval(1) self.slider.setMinimum(0) self.slider.setMaximum(100) self.slider.valueChanged.connect(self.changedValue) self.label = QLabel("0") self.label.setFont(QtGui.QFont("Sanserif", 15)) hbox.addWidget(self.slider) hbox.addWidget(self.label) self.setLayout(hbox) self.show() def changedValue(self): size = self.slider.value() self.label.setText(str(size)) App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
Run the complete code and this will be the result
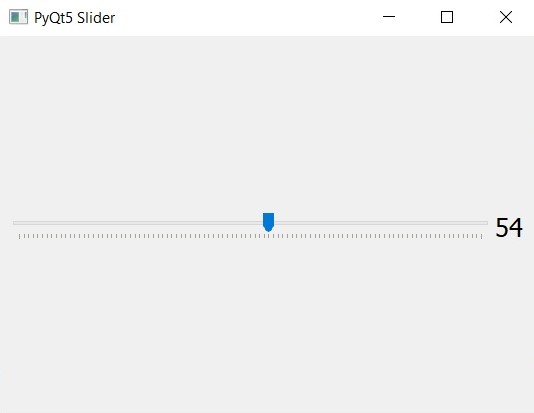
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email