In this PyQt5 tutorial i want to show you How To Create QComboBox In PyQt5. ComboBox provides a dropdown menu attached to a button, providing a list of options of which one can be selected by the user.
What is PyQt5 QComboBox ?
PyQt5 QComboBox is a widget in PyQt5 library that allows users to select from list of predefined options. it provides drop down list of items that can be selected by the user, and it is commonly used in graphical user interfaces (GUIs) to enable users to choose from set of options. QComboBox widget is a combination of QLineEdit widget and QListView widget. QLineEdit widget provides the text entry area where users can enter their own text, while the QListView widget provides drop down list of predefined items.
some of the common properties of the QComboBox widget include the number of items in the list, the current selected item, the text of the selected item, and list of items in the combo box. QComboBox widget also emits signals when the user selects an item, changes the current index, or types text in the line edit. In result we can say QComboBox widget is powerful tool for creating user friendly GUIs in PyQt5.
Also you can read more Python GUI articles in the below links
- Kivy GUI Development Tutorials
- TKinter GUI Development Tutorials
- Psyide2 GUI Development
- wxPython GUI Development Tutorials
- PyQt5 GUI Development Tutorials
First we need some imports.
1 2 3 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QDialog, QComboBox, QVBoxLayout, QLabel import sys |
After that we are going to create our main window class that extends from QDialog and we initialize our window requirements in the constructor of that class also we have called our InitWindow() method in the class.
1 2 3 4 5 6 7 8 9 |
class Window(QDialog): def __init__(self): super().__init__() self.title = "PyQt5 Combo Box" self.top = 200 self.left = 500 self.width = 300 self.height = 100 self.InitWindow() |
This is for setting our window title, window icon and window geometry, also we created the object of QVBoxLayout in here. because we need to add our QComboBox in the QVBoxLyout.
1 2 3 4 |
self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) vbox = QVBoxLayout() |
In here we have created the object of QComboBox, and we have added some items to the combobox.
1 2 3 4 5 6 |
self.combo = QComboBox() self.combo.addItem("Python") self.combo.addItem("Java") self.combo.addItem("C++") self.combo.addItem("C#") self.combo.addItem("Ruby") |
Because we want to add functionality for our combobox. so we need to connect the currentTextChanged signal of QComboBox with the comboChanged() method or slot that we will create.
1 |
self.combo.currentTextChanged.connect(self.comboChanged) |
So i want when we select an item from combobox, the item should be written in a label. now we need to create a QLabel.
1 2 3 |
self.label = QLabel() self.label.setFont(QtGui.QFont("Sanserif", 15)) self.label.setStyleSheet('color:red') |
In here we have added our combobox and label in the vbox layout.
1 2 |
vbox.addWidget(self.combo) vbox.addWidget(self.label) |
And this is the method that we have connected with the combobox signal at the top. basically first we have get the item value from the combobox and after that we have set the value to the label.
1 2 3 |
def comboChanged(self): text = self.combo.currentText() self.label.setText("You Have Selected : " + text) |
Also every PyQt5 application must create an application object.
1 |
App = QApplication(sys.argv) |
Finally, we enter the mainloop of the application. The event handling starts from this point.
1 2 |
window = Window() sys.exit(App.exec()) |
Complete source code for QComboBox
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
from PyQt5 import QtGui from PyQt5.QtWidgets import QApplication, QDialog, QComboBox, QVBoxLayout, QLabel import sys class Window(QDialog): def __init__(self): super().__init__() self.title = "PyQt5 Combo Box" self.top = 200 self.left = 500 self.width = 300 self.height = 100 self.InitWindow() def InitWindow(self): self.setWindowIcon(QtGui.QIcon("icon.png")) self.setWindowTitle(self.title) self.setGeometry(self.left, self.top, self.width, self.height) vbox = QVBoxLayout() self.combo = QComboBox() self.combo.addItem("Python") self.combo.addItem("Java") self.combo.addItem("C++") self.combo.addItem("C#") self.combo.addItem("Ruby") self.combo.currentTextChanged.connect(self.comboChanged) self.label = QLabel() self.label.setFont(QtGui.QFont("Sanserif", 15)) self.label.setStyleSheet('color:red') vbox.addWidget(self.combo) vbox.addWidget(self.label) self.setLayout(vbox) self.show() def comboChanged(self): text = self.combo.currentText() self.label.setText("You Have Selected : " + text) App = QApplication(sys.argv) window = Window() sys.exit(App.exec_()) |
Run complete code and this will be the result
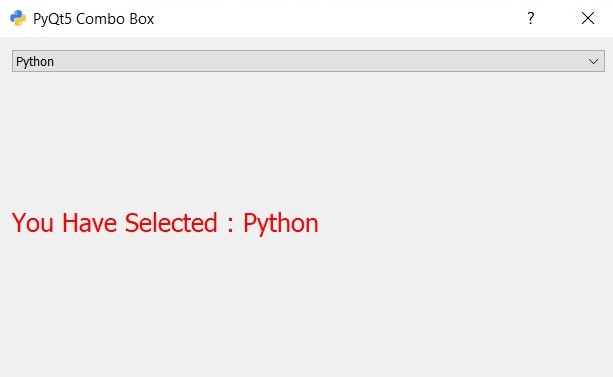
Also you can watch the complete video for this article.
Subscribe and Get Free Video Courses & Articles in your Email