In this Java tutorial we want to learn How to Create Java DatePicker with JavaFX,
so DatePicker control allows the user to enter a date as text or to select a date from a
calendar popup. The calendar is based on either the standard ISO-8601 chronology or any
of the other chronology classes.
Python GUI Development Tutorials with Video Training
- PyQt5 GUI Development Tutorials
- TKinter GUI Development Tutorials
- Pyside2 GUI Development Tutorials
- Kivy GUI Development Tutorials
- wxPython GUI Development Tutorials
This is the complete code for How to Create Java DatePicker with JavaFX
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.control.DatePicker; import javafx.scene.layout.HBox; import javafx.stage.Stage; import java.time.LocalDate; public class MyDatePicker extends Application { public static void main(String[] args) { launch(args); } @Override public void start(Stage stage) { stage.setTitle("JavaFX Date Picker - Codeloop.org"); HBox hbox = new HBox(); DatePicker datePicker = new DatePicker(); datePicker.setValue(LocalDate.of(20202, 9,5)); datePicker.setShowWeekNumbers(true); hbox.getChildren().add(datePicker); Scene scene = new Scene(hbox, 300,200); stage.setScene(scene); stage.show(); } } |
You can create javafx datepicker by creating the object of DatePicker class.
1 |
DatePicker datePicker = new DatePicker(); |
This is our HBox layout.
1 |
HBox hbox = new HBox(); |
You can set default date for java DatePicker. also i want to show week number for my DatePicker.
1 2 |
datePicker.setValue(LocalDate.of(20202, 9,5)); datePicker.setShowWeekNumbers(true); |
We need to add our datepicker in the hbox layout, if we don’t do this we will not see any
datepicker in javafx window, so it is important to add your any widget in a container or layout.
1 |
hbox.getChildren().add(datePicker); |
Also for every JavaFX application we need to create a Scene object. in the scene we need
to add our container with the width and height of the window, if you want to colorize your
window, you can do it in here. you can see that we have given 300 width and 200 height for the
window.
1 |
Scene scene = new Scene(hbox, 300,200); |
At the end you need to set your scene object to the stage of the window, and show the window.
1 2 |
stage.setScene(scene); stage.show(); |
Run the complete code and this is the result.
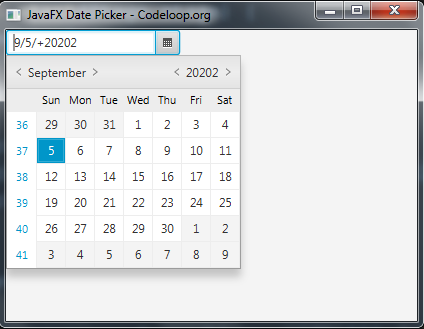
Subscribe and Get Free Video Courses & Articles in your Email