In this Python AWS lesson we want to learn How to Create AWS Access Key in Python, now first of all let’s talk about AWS Access Key.
What is AWS Access Key?
AWS access keys are credentials that allows you to access AWS services programmatically. They are commonly used by applications, codes and SDKs to make requests to AWS APIs securely. Access keys consist of two parts:
- Access Key ID: A unique identifier used to authenticate requests.
- Secret Access Key: A secret key used to sign requests and verify the authenticity of the request sender.
Prerequisites:
Before we start creating IAM user groups with Python and Boto3, we need to have following prerequisites:
-
-
- Python installed on your system.
- Boto3 library installed (pip install boto3).
- AWS credentials configured on your system (either through AWS CLI or environment variables).
-
Also make sure that you have already read these three articles, because they are related to this article.
- How to install Boto3 and AWS CLI for Python
- How to Configure AWS CLI to Use Boto3
- How to Create AWS IAM User with Python & Boto3
How to Create AWS Access Key in Python & Boto3:
Let’s talk about the steps involved in creating AWS access keys programmatically using Python and Boto3:
Step 1: Import Boto3
Start by importing the Boto3 library in your Python script:
1 |
import boto3 |
Step 2: Initialize Boto3 IAM Client
After that initialize the Boto3 IAM client to interact with AWS IAM (Identity and Access Management):
1 |
iam = boto3.client('iam') |
Step 3: Create AWS Access Key
Use the create_access_key method to generate a new set of AWS access keys:
1 2 3 |
response = iam.create_access_key() access_key_id = response['AccessKey']['AccessKeyId'] secret_access_key = response['AccessKey']['SecretAccessKey'] |
Step 4: Print Access Key Details
Print the access key ID and secret access key for future reference:
1 2 |
print("Access Key ID:", access_key_id) print("Secret Access Key:", secret_access_key) |
This is the complete code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
import boto3 # Initialize Boto3 IAM client iam = boto3.client('iam') # Specify IAM user name user_name = 'codeloop-updated' # Create AWS access key for the specified IAM user response = iam.create_access_key(UserName=user_name) access_key_id = response['AccessKey']['AccessKeyId'] secret_access_key = response['AccessKey']['SecretAccessKey'] # Print access key details print("Access Key ID:", access_key_id) print("Secret Access Key:", secret_access_key) |
Run the code and you will see this
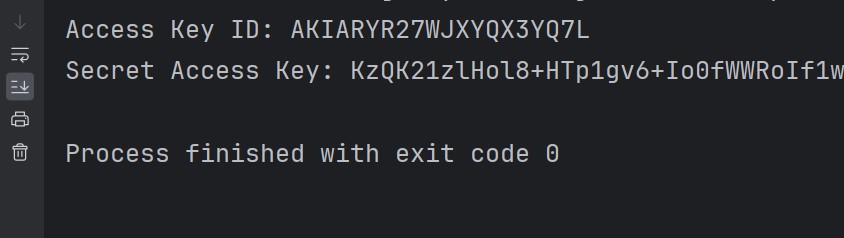
FAQs:
What are AWS access keys used for?
AWS access keys are used to authenticate programmatic requests to AWS services. They consist of an access key ID and a secret access key and are commonly used by applications, codes and SDKs to make requests to AWS APIs securely.
Can I create AWS access keys programmatically using Python?
Yes, you can create AWS access keys programmatically using Python and the Boto3 library, Boto3 is official AWS SDK for Python. create_access_key method in the IAM client of Boto3 allows you to generate new access keys.
How do I securely handle AWS access keys in my Python code?
It’s essential to handle AWS access keys securely to prevent unauthorized access to your AWS resources. You need to avoid hardcoding access keys in your code or storing them in plaintext files. Instead, consider using environment variables or AWS Secrets Manager to securely manage access keys.
Subscribe and Get Free Video Courses & Articles in your Email