In this lesson we want to learn How to Build Python GUI Application With wxPython. so first of all let’s talk about some concepts.
What is Python GUI ?
Python GUI or Graphical User Interface is a visual interface that a user interacts with that. It’s a type of interface that allows users to interact with application through graphical elements, such as buttons, text boxes, and drop-down menus instead of command-line interface.
In Python programming there are several libraries available that allow you to create GUI applications, such as Tkinter, wxPython, PyGTK, PyQt and others. these libraries provide a way to design the graphical interface, handle user input, and interact with the underlying application logic.
Building GUI in Python improves the user experience and make your application more accessible to more users, as we know that everyone is not comfortable with a command-line interface.
Popular Python GUI Frameworks ?
There are several popular Python GUI frameworks that you can use to build your applications. these are some of the most commonly used ones:
- Tkinter: Tkinter is standard GUI library for Python and it is included with most Python installations. It provides a simple way to create GUI applications, and it is good for small to medium sized projects, and you don’t need to install this.
- wxPython: wxPython is GUI toolkit that provides native look and feel on multiple platforms, including Windows, MacOS and Linux. it offers different features like support for widgets, dialogs and many more.
- PyGTK: PyGTK is set of Python bindings for the GTK+ GUI toolkit. it provides a rich set of GUI elements, and it is used by many popular open-source projects, such as GIMP and GNOME.
- PyQt: PyQt is set of Python bindings for Qt GUI toolkit. It’s known for its powerful features, and it is used by many large enterprises and commercial software products.
- Pygame: Pygame is a library for creating games and multimedia applications. It provides a simple way to create graphical elements, handle user input and create animations.
- Kivy: Kivy is an open-source library for creating cross-platform GUI applications. It’s especially good for mobile app development and provides support for touch inputs and gestures.
Each of these GUI frameworks has its own strengths and weaknesses, so the best one for your project will depend on your specific needs and requirements. and it is good exploring a few of them to find the one that best fits your needs.
What is wxPython ?
wxPython is cross-platform GUI toolkit for Python programming language. using wxPython you can create graphical user interfaces (GUIs) for desktop and mobile applications in easy way.
wxPython is built on top of wxWidgets C++ library and provides Python bindings for the library’s functionality. it means that wxPython applications can run on multiple platforms, including Windows, MacOS, and Linux and also it has native look and feel on each platform.
wxPython provides different features for GUI development, including support for buttons, text boxes, dialogs and many more. wxPython also provides an event-driven programming model that makes it easy to respond to user interactions and update the GUI dynamically. you can use wxPython in both commercial and open-source projects.
How to Install wxPython in Windows?
You can use pip for wxPython installation, open your command prompt or terminal and write this command.
1 |
brew install wxpython |
How to Install wxPython in Mac?
To install wxPython on MacOS, you can use the Homebrew package manager. To do this, open a terminal window and run the following command:
1 |
brew install wxpython |
How to Install wxPython in Linux?
For installing wxPython in Linux, you can use package manager for your distribution. For example, on Ubuntu, you can run the following command in a terminal window:
1 |
sudo apt-get install python3-wxgtk4.0 |
How to Create Python Basic Window in wxPython?
Now let’s create a basic window in wxPython, this is our code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import wx class MyWindow(wx.Frame): def __init__(self): super().__init__(None, title="Codeloop - Window Example", size=(300, 200)) # Set window icon self.SetIcon(wx.Icon("codeloop.png")) if __name__ == "__main__": app = wx.App(False) frame = MyWindow() frame.Show() app.MainLoop() |
In this example:
- We have created custom class MyWindow, and this class inherits from wx.Frame, which represents a top level window.
- Inside __init__ method of MyWindow, we are calling constructor of the superclass (wx.Frame) using super().__init__().
- After that we pass None as the parent window, and it indicates that this window is a top level window with no parent.
- We set the title of the window and the initial size to 300×200 pixels using the title and size parameters of the wx.Frame constructor.
- We set the window icon using self.SetIcon(wx.Icon(“”)).
Run the code and this will be the result
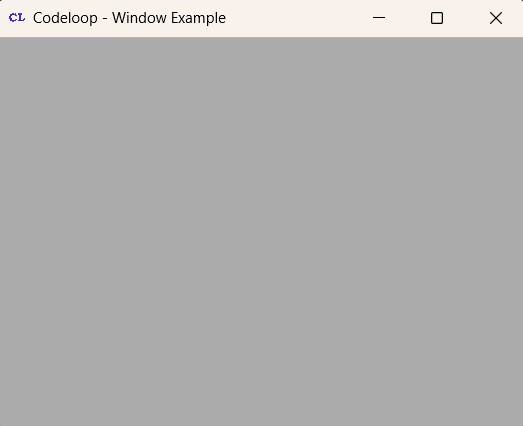
Widgets in wxPython
These are some of the most commonly used widgets in wxPython:
- wx.Button: It is a button that can be clicked to perform an action.
- wx.CheckBox: it is checkbox that can be checked or unchecked to indicate the state of a boolean option.
- wx.Choice: wxPython dropdown menu allows users to select one item from a list of choices.
- wx.TextCtrl: A text box that allows the user to enter text.
- wx.RadioButton: A radio button that allows the user to select one item from a group of mutually exclusive options.
- wx.StaticText: A non-editable text label.
- wx.ListBox: A list box that allows the user to select one or more items from a list.
- wx.ComboBox: A combination of a text box and a dropdown menu that allows the user to either type a value or select one from a list.
- wx.Slider: A slider that allows the user to select a value within a range by dragging a thumb.
- wx.Gauge: A progress bar that indicates the progress of a task.
Python Button in wxPython?
Let’s create a button using wxPython.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
import wx class MyWindow(wx.Frame): def __init__(self): super().__init__(None, title="Codeloop - Button Example", size=(300, 200)) # Set window icon self.SetIcon(wx.Icon("codeloop.png")) # Create a panel to hold the button panel = wx.Panel(self) # Create a button self.button = wx.Button(panel, label="Click Me") self.button.Bind(wx.EVT_BUTTON, self.on_button_click) # Bind button click event to a handler # Add the button to a sizer sizer = wx.BoxSizer(wx.VERTICAL) sizer.Add(self.button, 0, wx.ALIGN_CENTER | wx.ALL, 20) panel.SetSizer(sizer) def on_button_click(self, event): wx.MessageBox("Do you like codeloop.org website?", "Info", wx.OK | wx.ICON_INFORMATION) if __name__ == "__main__": app = wx.App(False) frame = MyWindow() frame.Show() app.MainLoop() |
In this example:
- We have created a custom class MyWindow that inherits from wx.Frame, and it represents the main window of the application.
- Inside __init__ method, we set up the window title, size and icon similar to the previous example.
- After that we creates a panel to hold the button. Panels are used to organize and group widgets in a window.
- Then we creates a button (self.button) with a label and bind its click event to the on_button_click method using Bind.
- In the on_button_click method, we display a message box (wx.MessageBox).
Run the complete code and this will be the result
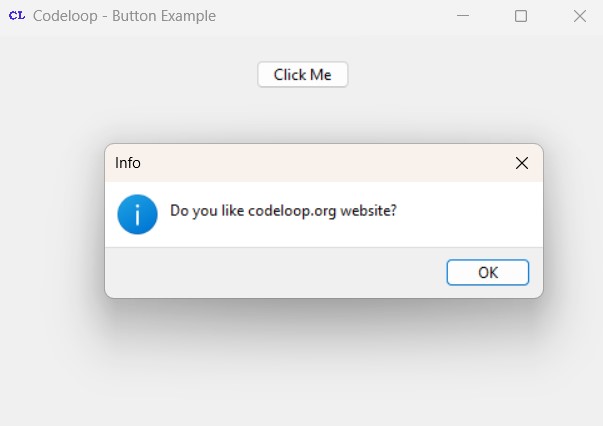
Python CheckBox in wxPython?
Now let’s learn how to create checkbox in wxPython, so checkbox is a graphical user interface element that allows users to toggle between two states: checked and unchecked. It typically appears as a small box next to a label, and when we click, it changes its state from checked to unchecked or vice versa.
This is our code
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
import wx class MyWindow(wx.Frame): def __init__(self): super().__init__(None, title="Codeloop - Checkbox Example", size=(300, 200)) # Set window icon self.SetIcon(wx.Icon("codeloop.png")) # Create a panel to hold the checkbox panel = wx.Panel(self) # Create a checkbox self.checkbox = wx.CheckBox(panel, label="Check Me") self.checkbox.Bind(wx.EVT_CHECKBOX, self.on_checkbox_toggle) # Bind checkbox toggle event to a handler # Add the checkbox to a sizer sizer = wx.BoxSizer(wx.VERTICAL) sizer.Add(self.checkbox, 0, wx.ALIGN_CENTER | wx.ALL, 20) panel.SetSizer(sizer) def on_checkbox_toggle(self, event): if self.checkbox.IsChecked(): wx.MessageBox("Checkbox Checked", "Info", wx.OK | wx.ICON_INFORMATION) else: wx.MessageBox("Checkbox Unchecked", "Info", wx.OK | wx.ICON_INFORMATION) if __name__ == "__main__": app = wx.App(False) frame = MyWindow() frame.Show() app.MainLoop() |
This example creates wxPython application with a checkbox. It consists of a custom class called MyWindow, and it inherits from wx.Frame. In the __init__ method, we set up window title, size and icon. after that we creates a panel to hold the checkbox. Also we have bounded the checkbox toggle event to on_checkbox_toggle method using Bind. In the on_checkbox_toggle method, we have a message box with different messages based on whether the checkbox is checked or unchecked.
Run the code and this will be the result
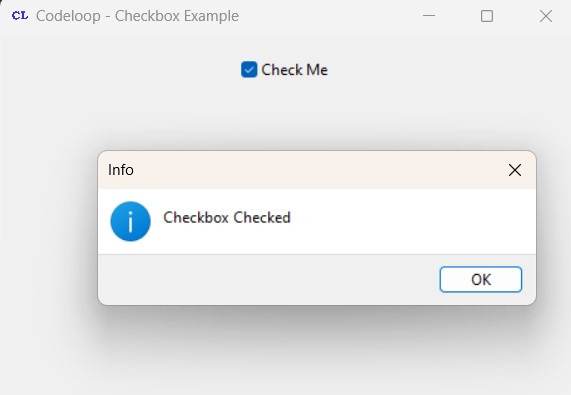
Python ComboBox in wxPython?
Now let’s create combobox in wxPython, so a ComboBox, also known as a drop down list or a combo box, it is a graphical user interface element commonly used in applications to present users with a list of options from which they can select one.
This is the example of combobox in wxPython
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
import wx class MyWindow(wx.Frame): def __init__(self): super().__init__(None, title="Codeloop - ComboBox Example", size=(300, 200)) # Set window icon self.SetIcon(wx.Icon("codeloop.png")) # Create a panel to hold ComboBox panel = wx.Panel(self) # Create a list of choices for the ComboBox choices = ["Python", "Java", "C++"] # Create a ComboBox self.combobox = wx.ComboBox(panel, choices=choices, style=wx.CB_DROPDOWN) self.combobox.Bind(wx.EVT_COMBOBOX, self.on_combobox_select) # Bind ComboBox select event to a handler # Add ComboBox to sizer sizer = wx.BoxSizer(wx.VERTICAL) sizer.Add(self.combobox, 0, wx.ALIGN_CENTER | wx.ALL, 20) panel.SetSizer(sizer) def on_combobox_select(self, event): selected_item = self.combobox.GetValue() wx.MessageBox(f"Selected item: {selected_item}", "Info", wx.OK | wx.ICON_INFORMATION) if __name__ == "__main__": app = wx.App(False) frame = MyWindow() frame.Show() app.MainLoop() |
In the above example, we creates a custom class MyWindow, and it inherits from wx.Frame, representing the main window of the application. Inside __init__ method, we set up window title, size and icon. Also we have a panel for holding the ComboBox. After that we have defined a list of choices (choices) for the ComboBox.
Run the code and this will be the result
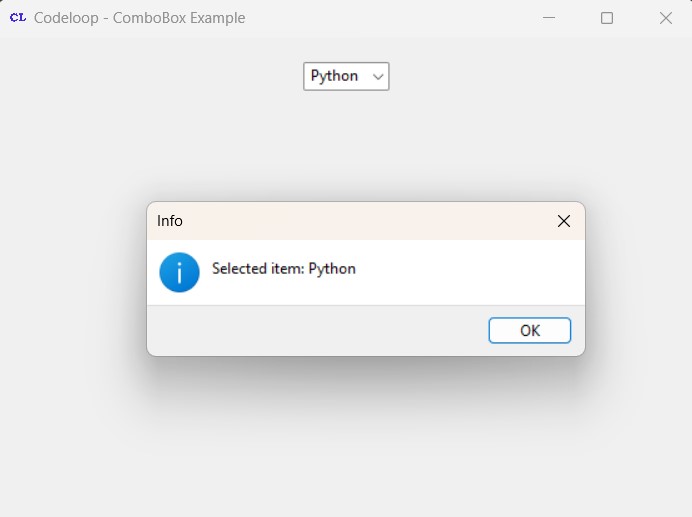
Subscribe and Get Free Video Courses & Articles in your Email