In this Python Game Development we want to learn about Game Development with Python & PyGame, so when you want to build games using Python, there different libraries and choices for you, one of the are PyGame, now first of all let’s talk about this library and after that we are going to create an example.
Introduction to PyGame
Pygame is popular and powerful library for game development in Python. because it is simple and it has user friendly interface, Pygame is easy, and beginners can create their own games easily. in this article we are going to talk about the process of installing Pygame, creating your first game and running it.
Getting Started
Before we start creating our game in Pygame, we need to install it. the easiest way to install Pygame is by using the pip, open your terminal or command prompt and write this command.
1 |
pip install pygame |
Key Features of Pygame
Pygame is cross platform library for writing video games. these are some key features:
- Easy to use API: Pygame has simple and easy API, and it is easy for beginners to get started with game development.
- Multi-platform support: Pygame runs on Windows, macOS and Linux and its code is portable, it means that you can develop games that can be played on multiple platforms.
- Graphics and Sound: Pygame provides basic graphics and sound functionality, and it is easy to create 2D games and simple animations.
- Fast and Lightweight: Pygame is fast and lightweight and it is good for developing games with limited system resources or for use on mobile devices.
- Large Community: Pygame has large and active community of developers and it provides support and resources for beginners and experienced developers.
- Free and Open-Source: Pygame is free and open source, and that is accessible to anyone and allowing for modifications and customization of the code.
Game Development with Python & PyGame
Creating Your First Game: after you have installed Pygame, you are ready to create your first game. Pygame provides different tools and resources to help you build your game, including game loop, sprites and graphics. In this article we will create a simple game that involves bouncing ball.
The Game Loop: game loop is the backbone of any game in Pygame. it is responsible for handling events and updating the screen. the game loop runs continuously until the player closes the game.
Sprites: Sprites are the individual graphics or objects that make up your game. in our bouncing ball game, the ball is sprite. you can use sprites to add interactivity to your game such as collisions and animations.
Graphics: Pygame provides different graphics functions to help you create and display graphics in your game. In this article we will use the draw.circle function to create the ball.
Running Your Game: Once you have created your game, you’re ready to run it. Simply run the Python code in your terminal or command prompt, and your game should start.
Basic Example of Pygame
This is a simple game example in Pygame:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
import pygame # Initialize Pygame pygame.init() # Set screen dimensions screen = pygame.display.set_mode((400, 300)) # Run game loop running = True while running: # Handle events for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Clear screen screen.fill((255, 255, 255)) # Draw rectangle pygame.draw.rect(screen, (255, 0, 0), (50, 50, 100, 100)) # Update the screen pygame.display.update() # Quit Pygame pygame.quit() |
This code creates Pygame window with white background and red rectangle. the game loop runs until the user closes the window, and pygame.draw.rect() function is used to draw the rectangle on the screen.pygame.display.update() function is used to update the screen with any changes made to it.
Run the code and this will be the result
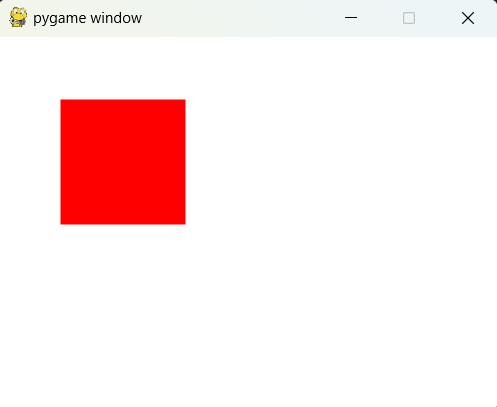
Subscribe and Get Free Video Courses & Articles in your Email