In this Flask Tutorial we are going to to learn Creating Flask Flash Messages, so good
applications and user interfaces are all about feedback. If the user does not get enough
feedback they will probably end up hating the application. Flask provides a really simple
way to give feedback to a user with the flashing system. The flashing system basically makes
it possible to record a message at the end of a request and access it next request and only next
request. This is usually combined with a layout template that does this. Note that browsers
and sometimes web servers enforce a limit on cookie sizes. This means that flashing messages
that are too large for session cookies causes message flashing to fail silently.
OK we are going to use our Flask Project from the previous article, where we have a login
form, so now i want when a user enter wrong password or username, i want to show error
in Flask Flash Messages.
So this is our forms.py file, and in this file we have created our simple login form and you
can see that we have added some ready validators from Flask-WTF that is InputRequired().
For more information about Flask-WTF you can read this article Flask Forms with Flask-WTF.
1 2 3 4 5 6 7 8 9 10 |
from flask_wtf import FlaskForm from wtforms import StringField, PasswordField from wtforms.validators import InputRequired class LoginForm(FlaskForm): username = StringField('Username', validators=[InputRequired()]) password = PasswordField('Password', validators=[InputRequired()]) |
And this is our app.py file with our required view functions and you can see that we have also
added our login view function in here. so in the Login view function we need to write our Flask
Flash Messages, till now we haven’t learned about database functionality in Flask, we are going
to just manually check the username and password. make sure that you have imported the flash.
1 2 3 4 5 6 7 |
if form.validate_on_submit(): if request.form['username'] != 'codeloop' or request.form['password'] != '12345': flash("Invalid Credentials, Please Try Again") else: return redirect(url_for('index')) |
So now this is the complete code for app.py file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
from flask import Flask, render_template, flash, request, redirect, url_for from forms import LoginForm #create the object of Flask app = Flask(__name__) app.config['SECRET_KEY'] = 'hardsecretkey' #creating our routes @app.route('/') def index(): return render_template('index.html') #login route @app.route('/login' , methods = ['GET', 'POST']) def Login(): form = LoginForm() if form.validate_on_submit(): if request.form['username'] != 'codeloop' or request.form['password'] != '12345': flash("Invalid Credentials, Please Try Again") else: return redirect(url_for('index')) return render_template('login.html', form = form) #run flask app if __name__ == "__main__": app.run(debug=True) |
This is our base.html, we have already talked about creating templates in Flask, you can
read this article Introduction to Flask Templates. you need to just create templates folder
in your working directory.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>{% block title %} {% endblock %}</title> <!-- CSS Bootstrap CDN Link --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous"> </head> <body> <nav class="navbar navbar-expand-lg navbar-dark bg-dark"> <div class="container"> <a class="navbar-brand" href="{{url_for('index')}}">CodeLoop</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav mr-auto"> <li class="nav-item active"> <a class="nav-link" href="{{url_for('index')}}">Home <span class="sr-only">(current)</span></a> </li> <li class="nav-item"> <a class="nav-link" href="#">Logout</a> </li> </ul> <form class="form-inline my-2 my-lg-0"> <input class="form-control mr-sm-2" type="search" placeholder="Search" aria-label="Search"> <button class="btn btn-outline-success my-2 my-sm-0" type="submit">Search</button> </form> </div> </div> <a href="{{url_for('Login')}}"><button class="btn btn-success navbar-btn">Login</button> </a> <a href=""><button class="btn btn-success navbar-btn">Signup</button> </a> </nav> <!-- JS, Popper.js, and jQuery --> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js" integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js" integrity="sha384-OgVRvuATP1z7JjHLkuOU7Xw704+h835Lr+6QL9UvYjZE3Ipu6Tp75j7Bh/kR0JKI" crossorigin="anonymous"></script> {% block body %} {% endblock %} </body> </html> |
we are going to show our Flask Flash Messages in login.html, so you can use this code for
showing the Flash Messages. right now there is no design for the Flash Message, but we will
do that.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
{% with messages = get_flashed_messages() %} {% if messages %} {% for message in messages %} {{message}} {% endfor %} {% endif %} {% endwith %} |
OK now this is the complete code for our login.html.
templates/login.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 |
{% extends 'base.html' %} {% block title %} Home {% endblock %} {% block body %} <div class="container"> <h1>Home Page - Welcome to codeloop.org</h1> <h3>Tutorial Number 10 </h3> <br> <br> <hr> {% with messages = get_flashed_messages() %} {% if messages %} {% for message in messages %} {{message}} {% endfor %} {% endif %} {% endwith %} <h1>Please Login</h1> <form action="" method="post" novalidate> {{form.csrf_token}} <p> {{form.username.label}} {{form.username(size=32)}} {% for error in form.username.errors %} <span style="color:red;"> {{error}} </span> {% endfor %} </p> <p> {{form.password.label}} {{form.password(size=32)}} {% for error in form.password.errors %} <span style="color:red;"> {{error}} </span> {% endfor %} </p> <p> <input type="submit" value="Login" class="btn btn-success"> </p> </form> </div> {% endblock %} |
And this is our index.html file.
templates/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
{% extends 'base.html' %} {% block title %} Home {% endblock %} {% block body %} <div class="container"> <h1>Home Page - Welcome to codeloop.org</h1> <h3>Tutorial Number 10 </h3> <p> In this tutorial we are going to talk about Flask Flash Messages. </p> </div> {% endblock %} |
Now run the project and go to http://localhost:5000/login, if you enter a wrong username
and password, you will see a Flash Message.
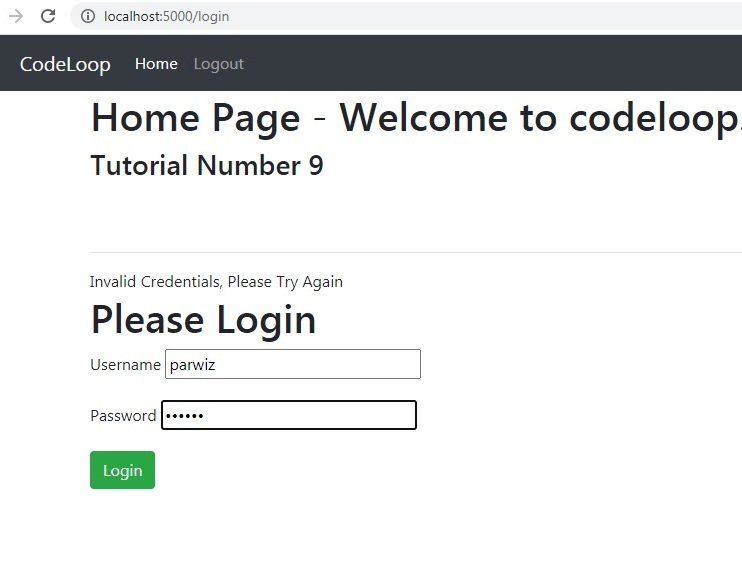
Adding Bootstrap Styles to Flash Message
So our code is working, but the Flash Message is ugly, we need to add some bootstrap design
for the Flash Message. so now this is the new code for Flash Message. we have just added a
simple design.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
{% with messages = get_flashed_messages() %} {% if messages %} {% for message in messages %} <div class="alert alert-success alert-dismissable" role="alert"> <button type="button" class="close" data-dismiss="alert" aria-label="close"> <span aria-hidden="true">X</span> </button> {{message}} </div> {% endfor %} {% endif %} {% endwith %} |
Now This is the complete code for login.html.
templates/login.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 |
{% extends 'base.html' %} {% block title %} Home {% endblock %} {% block body %} <div class="container"> <h1>Home Page - Welcome to codeloop.org</h1> <h3>Tutorial Number 10 </h3> <br> <br> <hr> {% with messages = get_flashed_messages() %} {% if messages %} {% for message in messages %} <div class="alert alert-success alert-dismissable" role="alert"> <button type="button" class="close" data-dismiss="alert" aria-label="close"> <span aria-hidden="true">X</span> </button> {{message}} </div> {% endfor %} {% endif %} {% endwith %} <h1>Please Login</h1> <form action="" method="post" novalidate> {{form.csrf_token}} <p> {{form.username.label}} {{form.username(size=32)}} {% for error in form.username.errors %} <span style="color:red;"> {{error}} </span> {% endfor %} </p> <p> {{form.password.label}} {{form.password(size=32)}} {% for error in form.password.errors %} <span style="color:red;"> {{error}} </span> {% endfor %} </p> <p> <input type="submit" value="Login" class="btn btn-success"> </p> </form> </div> {% endblock %} |
Now run the project and go to http://localhost:5000/login, if you enter a wrong username
and password, you will see a nice Flash Message.
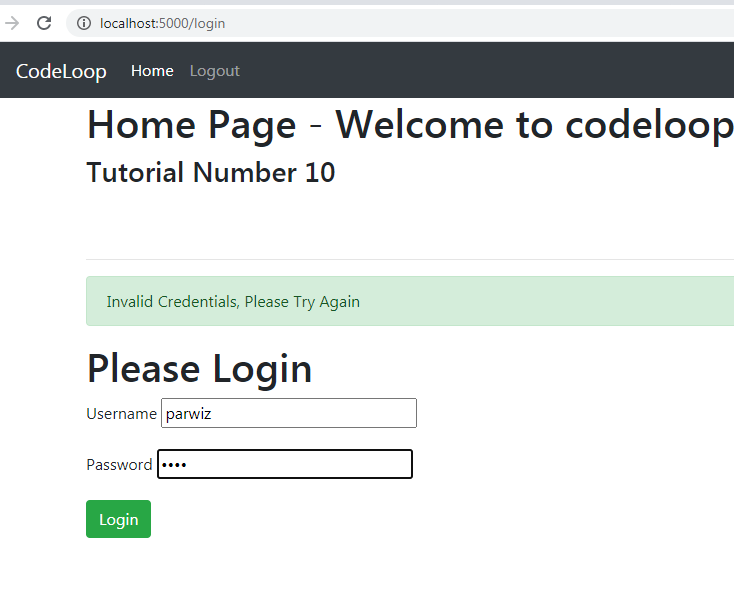
Also you can watch my complete 4 hours training on Flask Web Development
Subscribe and Get Free Video Courses & Articles in your Email