In this Flask Tutorial, i want to show you Adding Bootstrap Styles to Flask. so there are
three different ways that you can add Bootstrap Styles in your Flask Application, that we will learn one by one.
If you are interested in Web Development with Django Framework, than you can read the
complete articles in this link, Django Web Development Tutorials.
1: Adding Bootstrap Files
the first way is that you can just create static folder in your Flask Application
working directory, download the bootstrap from getbootstrap.com, and add the files in the
static folder, and after that you need to link the CSS and JS files in your base.html.
So first of all this is our app.py file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
from flask import Flask, render_template #create the object of Flask app = Flask(__name__) #creating our routes @app.route('/') def index(): data = "Codeloop" return render_template('index.html', data = data) #contact routes @app.route('/contact') def Contact(): return render_template('contact.html') #run flask app if __name__ == "__main__": app.run(debug=True) |
templates/base.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>{% block title %} {% endblock %}</title> <link href="{{url_for('static', filename = 'css/bootstrap.min.css')}}" rel="stylesheet"> </head> <body> <nav class="navbar navbar-expand-lg navbar-dark bg-dark"> <div class="container"> <a class="navbar-brand" href="#">CodeLoop</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav mr-auto"> <li class="nav-item active"> <a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a> </li> <li class="nav-item"> <a class="nav-link" href="#">Contact</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Logout</a> </li> </ul> <form class="form-inline my-2 my-lg-0"> <input class="form-control mr-sm-2" type="search" placeholder="Search" aria-label="Search"> <button class="btn btn-outline-success my-2 my-sm-0" type="submit">Search</button> </form> </div> </div> <a href=""><button class="btn btn-success navbar-btn">Login</button> </a> <a href=""><button class="btn btn-success navbar-btn">Signup</button> </a> </nav> {% block body %} {% endblock %} <script src="{{url_for('static', filename='js/bootstrap.min.js')}}"></script> </body> </html> |
You can see that in our base.html, we have linked our bootstrap CSS and Javascript
using this code. we have linked the CSS at the top and the Javascript at the bottom.
It is always good to add your Javascript at the bottom. and also we have added a simple
Navbar code from Bootstrap website.
1 |
<link href="{{url_for('static', filename = 'css/bootstrap.min.css')}}" rel="stylesheet"> |
1 |
<script src="{{url_for('static', filename='js/bootstrap.min.js')}}"></script> |
templates/index.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
{% extends 'base.html' %} {% block title %} Home {% endblock %} {% block body %} <div class="container"> <h1>Home Page - Welcome to codeloop.org</h1> <h3>Tutorial Number 5 </h3> <p> In this tutorial we are going to talk about flask Adding Bootstrap Styles. </p> <h1>Welcome to . {{data}}</h1> </div> {% endblock %} |
templates/contact.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
{% extends 'base.html' %} {% block title %}Contact {% endblock %} {% block body %} <div class="container"> <h1>Contact Page - Welcome to codeloop.org</h1> <h3>Tutorial Number 5 </h3> <p> In this tutorial we are going to talk about flask Adding Bootstrap Styles. </p> </div> {% endblock %} |
Now run the the Project and go to http://localhost:5000/, this will be the result.
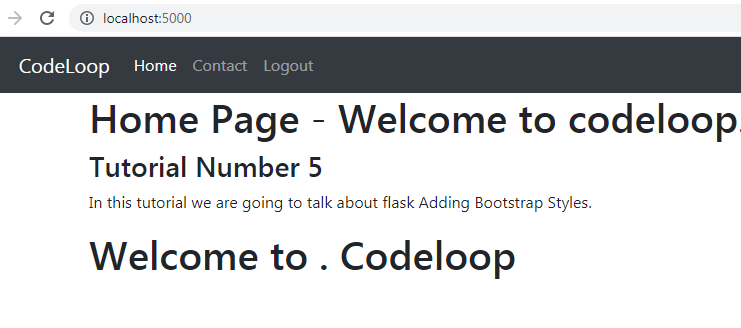
2: Adding Bootstrap Library
The second way is using Flask-Bootstrap library, Flask-Bootstrap packages Bootstrap into an extension that mostly consists of a blueprint named ‘bootstrap’. It can also create links to serve Bootstrap from a CDN and works with no boilerplate code in your application.
So first of all you need to install Flask-Bootstrap, you can use pip for the installation.
1 |
pip install Flask-Bootstrap |
After the installation , you need to first import Flask-Bootstrap.
1 |
from flask_bootstrap import Bootstrap |
After that you can simply add that to your flask app.py.
1 |
bootstrap = Bootstrap(app) |
3: Adding Bootstrap CDN Links
The third way is so simple, you need to just copy the BootstrapCDN links from Bootstrap Website, and after that add the links for CSS, Javascript and others in your base.html like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>{% block title %} {% endblock %}</title> <!-- CSS Bootstrap CDN Link --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/css/bootstrap.min.css" integrity="sha384-9aIt2nRpC12Uk9gS9baDl411NQApFmC26EwAOH8WgZl5MYYxFfc+NcPb1dKGj7Sk" crossorigin="anonymous"> </head> <body> <nav class="navbar navbar-expand-lg navbar-dark bg-dark"> <div class="container"> <a class="navbar-brand" href="#">CodeLoop</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> <div class="collapse navbar-collapse" id="navbarSupportedContent"> <ul class="navbar-nav mr-auto"> <li class="nav-item active"> <a class="nav-link" href="#">Home <span class="sr-only">(current)</span></a> </li> <li class="nav-item"> <a class="nav-link" href="#">Contact</a> </li> <li class="nav-item"> <a class="nav-link" href="#">Logout</a> </li> </ul> <form class="form-inline my-2 my-lg-0"> <input class="form-control mr-sm-2" type="search" placeholder="Search" aria-label="Search"> <button class="btn btn-outline-success my-2 my-sm-0" type="submit">Search</button> </form> </div> </div> <a href=""><button class="btn btn-success navbar-btn">Login</button> </a> <a href=""><button class="btn btn-success navbar-btn">Signup</button> </a> </nav> <!-- JS, Popper.js, and jQuery --> <script src="https://code.jquery.com/jquery-3.5.1.slim.min.js" integrity="sha384-DfXdz2htPH0lsSSs5nCTpuj/zy4C+OGpamoFVy38MVBnE+IbbVYUew+OrCXaRkfj" crossorigin="anonymous"></script> <script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js" integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.5.0/js/bootstrap.min.js" integrity="sha384-OgVRvuATP1z7JjHLkuOU7Xw704+h835Lr+6QL9UvYjZE3Ipu6Tp75j7Bh/kR0JKI" crossorigin="anonymous"></script> {% block body %} {% endblock %} </body> </html> |
Now if you run your Flask Project , the result is the same.
http://localhost:5000/
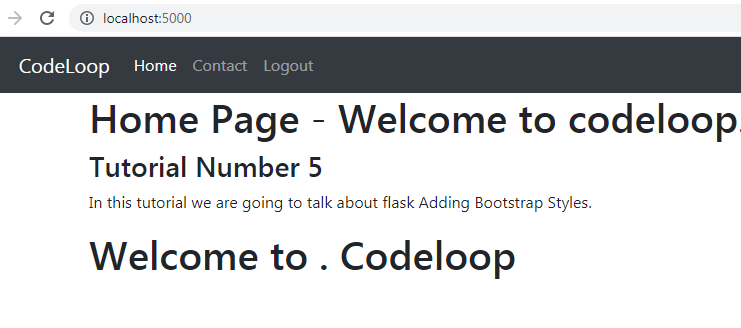
http://localhost:5000/contact
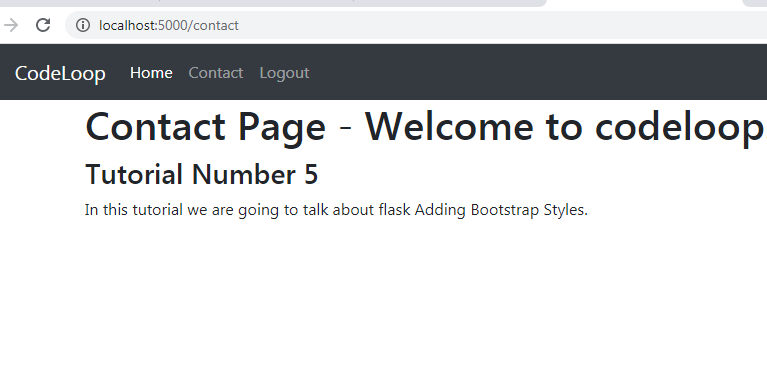
Subscribe and Get Free Video Courses & Articles in your Email