In this Android Studio article we are going to talk about
Facebook Audience Network Interstitial Ads Integration, so in the previous
article we have talked about Banner Ads Integration In Android Studio . if you are an
android developer you will be familiar with Admob, and the FAN has the same process
as Admob, and you can monetize your android application with Facebook Audience Network.
also for good explanation you can watch the video for this at the end of the article.
Also you can read more android development articles
1: Android Development Articles
Also you can check Python GUI Development Tutorials in the below link.
1: PyQt5 GUI Development Tutorials
2: TKinter GUI Development Tutorials
3: Pyside2 GUI Development Tutorials
4: Kivy GUI Development Tutorials
5: wxPython GUI Development Tutorials
What Is Facebook Audience Network (FAN) ?
Audience Network allows advertisers to extend Facebook and Instagram campaigns
across the internet – onto thousands of high-quality websites and apps.
People spend a lot of their time on Facebook and Instagram. But they are also spending
time on other apps and sites. Audience Network helps advertisers reach more of the people
they care about in the other places where they’re spending their time.
In a Facebook ad campaign study, conversion rates were 8x higher among people who
saw ads across Facebook, Instagram and Audience Network than people who only saw
the ads on Facebook. Audience Network ads use the same targeting, auction, delivery
and measurement systems as Facebook ads.
Supported Objectives
Audience Network currently supports the following objectives:
-
Brand awareness (video only)
-
Reach (video only)
-
Traffic
-
Engagement (video only)
-
App installs
-
Video views (video only)
-
Conversions
-
Catalog sales
The behavior of an ad after it’s clicked on depends on the type of ad objective an advertiser chooses. When someone clicks on an ad, depending on the ad objective, what will happen is:
-
The ad will open a link in a new browser window
-
The ad will prompt someone to install an app
-
The ad will launch an existing app on their mobile device
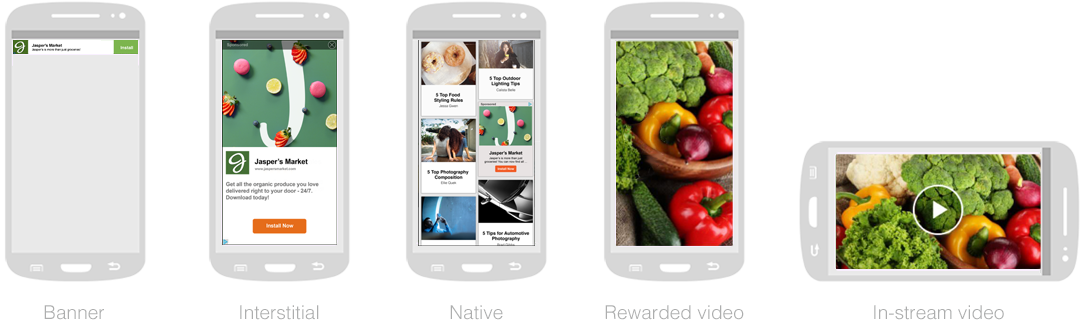
Implementation Of FAN Interstitial Ads In Android Studio
So now we are going to talk about Interstitial Ads implementation of Facebook Audience
Network in Android Studio.
1: OK first of all you need to create a New Project in Android Studio
2: After that you need to include the SDK for Facebook Audience Network, open build.gradle(Module:app), and in the dependencies section you need to add
this line of code,
1 |
implementation 'com.facebook.android:audience-network-sdk:5.+' |
and after that sync your project, also in your Manifest.xml file you need to add internet permission.
1 |
<uses-permission android:name="android.permission.INTERNET"/> |
3: Before creating an ad object and loading ads, you should initialize the Audience Network SDK.
It is recommended to do this at app launch.
1 2 3 4 5 6 7 8 9 10 |
public class YourApplication extends Application { ... @Override public void onCreate() { super.onCreate(); // Initialize the Audience Network SDK AudienceNetworkAds.initialize(this); } ... } |
4: Add the following code at the top of your Activity in order to import the Facebook Ads SDK.
1 |
import com.facebook.ads.*; |
5: Initialize the InterstitialAd
.
1 2 3 4 5 6 7 8 9 10 11 12 |
private InterstitialAd interstitialAd; @Override public void onCreate(Bundle savedInstanceState) { ... // Instantiate an InterstitialAd object. // NOTE: the placement ID will eventually identify this as your App, you can ignore it for // now, while you are testing and replace it later when you have signed up. // While you are using this temporary code you will only get test ads and if you release // your code like this to the Google Play your users will not receive ads (you will get a no fill error). interstitialAd = new InterstitialAd(this, "YOUR_PLACEMENT_ID"); ... |
6: Showing Interstitial Ads, set an intertitialAdListener, load the ad and show ad immediately
its loaded successfully.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
public class InterstitialAdActivity extends Activity { private final String TAG = InterstitialAdActivity.class.getSimpleName(); private InterstitialAd interstitialAd; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); // Instantiate an InterstitialAd object. // NOTE: the placement ID will eventually identify this as your App, you can ignore it for // now, while you are testing and replace it later when you have signed up. // While you are using this temporary code you will only get test ads and if you release // your code like this to the Google Play your users will not receive ads (you will get a no fill error). interstitialAd = new InterstitialAd(this, "YOUR_PLACEMENT_ID"); // Set listeners for the Interstitial Ad interstitialAd.setAdListener(new InterstitialAdListener() { @Override public void onInterstitialDisplayed(Ad ad) { // Interstitial ad displayed callback Log.e(TAG, "Interstitial ad displayed."); } @Override public void onInterstitialDismissed(Ad ad) { // Interstitial dismissed callback Log.e(TAG, "Interstitial ad dismissed."); } @Override public void onError(Ad ad, AdError adError) { // Ad error callback Log.e(TAG, "Interstitial ad failed to load: " + adError.getErrorMessage()); } @Override public void onAdLoaded(Ad ad) { // Interstitial ad is loaded and ready to be displayed Log.d(TAG, "Interstitial ad is loaded and ready to be displayed!"); // Show the ad interstitialAd.show(); } @Override public void onAdClicked(Ad ad) { // Ad clicked callback Log.d(TAG, "Interstitial ad clicked!"); } @Override public void onLoggingImpression(Ad ad) { // Ad impression logged callback Log.d(TAG, "Interstitial ad impression logged!"); } }); // For auto play video ads, it's recommended to load the ad // at least 30 seconds before it is shown interstitialAd.loadAd(); } } |
7: Finally, add the following code to your Activity’s onDestroy() function to release resources the InterstitialAd
uses:
1 2 3 4 5 6 7 |
@Override protected void onDestroy() { if (interstitialAd != null) { interstitialAd.destroy(); } super.onDestroy(); } |
Run the complete project and this will be the result
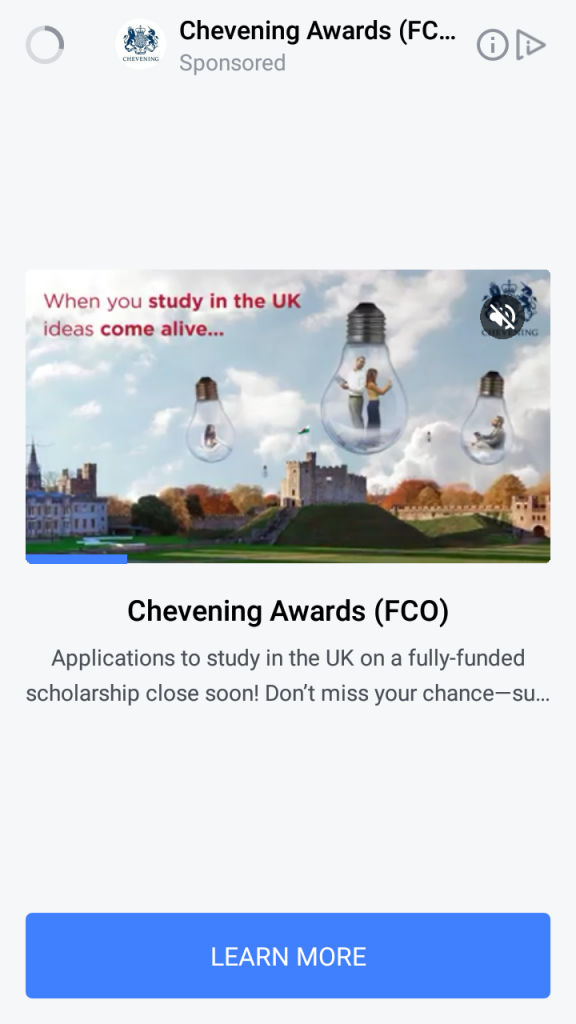
Also you can watch the complete video for this article
Subscribe and Get Free Video Courses & Articles in your Email
i want to learn android from scratch how do i start
you can learn from my youtube channel and also my this blog
I want to use both admob and Fan Interstitial, always i get errors after implementing admob interstitial Facebook interstitial refusing what should i do
you need to use ad mediation, can u tell me what is the error
Thanks for any other fantastic article. Where else may just anybody get that kind of info in such a perfect way of writing?
I’ve a presentation subsequent week, and I’m at the search for such info.
Look at my web site; Royal CBD
Simply desire to say your article is as astonishing.
The clearness for your post is simply nice and i can suppose
you’re knowledgeable on this subject. Fine along with
your permission let me to take hold of your feed to
keep updated with impending post. Thanks 1,000,000
and please continue the enjoyable work.
Your means of explaining everything in this paragraph is actually fastidious, all be capable of effortlessly know it, Thanks a lot. https://letpost.net/groups/furunculosis-footage-causes-symptoms-remedy/
I enjoy what you guys tend to be up too. This sort of clever work and coverage!
Keep up the wonderful works guys I’ve you guys to our blogroll.
Oh my goodness! Amazing article dude! Many thanks, However I
am experiencing problems with your RSS. I don’t understand the reason why I
cannot subscribe to it. Is there anybody else having identical RSS
problems? Anybody who knows the answer can you kindly
respond? Thanx!! http://ministerioprofetico.cl/congregarnos/
I did every thing and the code is working but the problem is what if i want the interstital show when i go to activity 1 or 2 or 3 how can i control that
you can check the facebook audience network documentation, they have some callback for interstitial ads