In this article we want to talk about Python Exception Types, Python is popular and high level programming language that is widely used by developers and data scientists. one of the important concept in Python is that how to handle errors and exceptions. in this article we want to talk about different types of Python exceptions and how to handle them.
Exception Types in Python
Python has different builtin exception types, so when an error occurs, these exceptions can be raised intentionally by programmer or by interpreter.
These are some of the most common exception types in Python:
- NameError: This exception occurs when you try to reference a variable or function that does not exists in the current namespace.
- TypeError: This exception occurs when you try to perform an operation on incompatible data types. for example if we want to to add a string and an integer, than it will raise TypeError exception.
- ValueError: This exception occurs when you try to perform an operation on a value that is the correct type but it is not a valid value. for example if you want to convert string hello to an integer than it will raise a ValueError.
- IndexError: This exception occurs when you try to access an index that is out of range for a sequence for example list or string.
- KeyError: This exception occurs when you try to access a dictionary key that does not exist.
- ZeroDivisionError: This exception occurs when you try to divide a number by zero.
For handling exceptions in Python, you can use try except block. so try block contains the code that might raise an exception, and except block contains the code that should be executed if an exception is raised. this is an example:
1 2 3 4 |
try: x = 1/0 except ZeroDivisionError: print("Cannot divide by zero") |
In the above example try block attempts to divide the number 1 by 0, and this will raise ZeroDivisionError exception. also we have wrapped the code in try except block, this program will not crash. but except block will execute and it will print a message.
If you run the code this will be the result
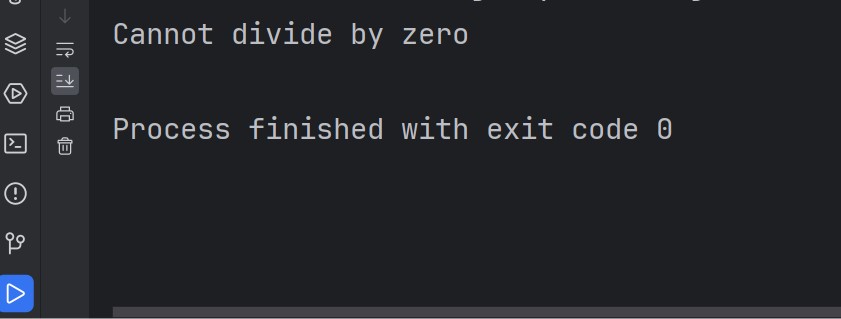
You can also catch multiple exceptions in single try except block and you can separate them with commas. this is an example:
1 2 3 4 |
try: x = int("hello") except (ValueError, TypeError): print("Could not convert string to integer") |
In the above example try block attempts to convert string hello to an integer, and it will raise ValueError or TypeError. because we have included both exceptions in the except block, this program will catch the error and print a message.
FAQs:
What are the three types of errors in Python?
- Syntax Errors: Occur when the parser detects an incorrect statement.
- Runtime Errors: Occur during the execution of a program and are also known as exceptions.
- Logical Errors: Occur when a program runs without crashing but produces incorrect results.
How many types of exception handling are there?
Python primarily uses three constructs for handling exceptions:
- try: block of code to be tested for errors.
- except: block of code that will be executed if an error occurs.
- finally: block of code that will be executed regardless of whether an exception occurs or not.
When to use exceptions in Python?
Exceptions should be used in Python to handle error conditions gracefully. Specifically, use exceptions when:
- You need to handle errors without stopping the execution of your program.
- You want to manage specific errors in different ways.
- You need to ensure that certain cleanup code runs no matter what
Which classes are exception classes in Python?
The primary base class for all exceptions in Python is the BaseException class. Other commonly used exception classes are:
- Exception: base class for all built-in, non-exit exceptions.
- ArithmeticError: base class for errors that occur for numeric operations.
- ZeroDivisionError
- OverflowError
- FloatingPointError
- LookupError: base class for errors raised when a lookup operation fails.
- IndexError
- KeyError
- EnvironmentError: base class for errors raised for outside the Python environment.
- IOError
- OSError
- Specific exceptions like ValueError, TypeError, NameError, AttributeError, ImportError, etc.
Subscribe and Get Free Video Courses & Articles in your Email