In this lesson we want to learn about Dictionary Methods in Python, first of all let’s talk about Python Dictionary.
What is Python Dictionary ?
Python dictionary is a collection of key value pairs, where each key is mapped to specific value. Dictionaries are also known as associative arrays or maps in other programming languages.
In Python, dictionaries are defined using curly braces {} and are composed of key value pairs separated by colons :. for example:
1 |
person = {'name': 'John', 'age': 32, 'gender': 'male'} |
In this example, ‘name’, ‘age’, and ‘gender’ are the keys, and ‘John’, 32, and ‘male’ are the values associated with those keys.
Dictionaries are unordered and mutable, it means that you can add, remove and modify elements in a dictionary after it has been created. they are also optimized for fast lookups, and this is useful for data that needs to be quickly retrieved based on specific key.
Python Dictionary Methods
Python dictionaries have several built in methods that allow you to perform common operations on dictionaries. some of the most commonly used dictionary methods include:
Method | Description |
---|---|
dict.clear() | Removes all items from the dictionary. |
dict.copy() | Returns shallow copy of the dictionary. |
dict.fromkeys(keys, value) | Returns new dictionary with the specific keys and each key mapped to the specified value. |
dict.get(key, default) | Returns value associated with specific key or the default value if the key is not found. |
dict.items() | Returns view of the dictionary’s (key, value) pairs as a list of tuples. |
dict.keys() | Returns view of the dictionary’s keys. |
dict.pop(key, default) | Removes specific key and returns its value or the default value if the key is not found. |
dict.update(other_dict) | Adds the items from other_dict to the dictionary, overwriting any existing values for matching keys. |
dict.values() | Returns a view of the dictionary’s values. |
This is an example of using some of the dictionary methods:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
# Define dictionary person = {'name': 'John', 'age': 32, 'gender': 'male'} # Use items() method to get a view of dictionary items items = person.items() print(list(items)) # Use keys() method to get view of the dictionary's keys keys = person.keys() print(list(keys)) # Use values() method to get view of dictionary's values values = person.values() print(list(values)) # Use get() method to retrieve value for key age = person.get('age', 0) print(age) |
In this example, we have defined a dictionary person with keys name, age and gender. After that we have used items(), keys(), and values() methods to get views of the dictionary’s items, keys, and values. And lastly we use get() method to retrieve the value associated with the ‘age’ key.
Run the code and this will be the result
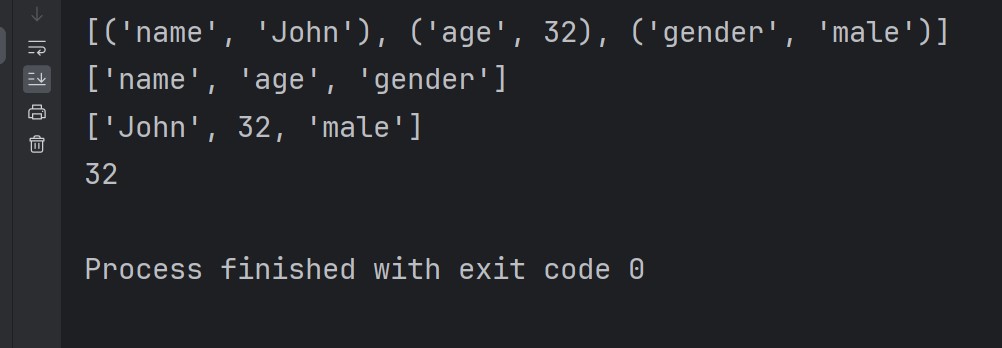
Subscribe and Get Free Video Courses & Articles in your Email