In this Android Studio article i want to show you How To Create PDF Book Application. so for good explanation you can watch the video for this article.
Also check my Android Development Courses
Now lets get started !
1: First of all create a new project in your Android Studio, and choose Empty Activity also iam using API 20 for this project.
2: OK after creating our project in Android Studio, you need to open build.gradle(Module:App) file and add this library in the dependency section. because this is the library that we are going to use for reading our pdf file.
1 |
implementation 'com.github.barteksc:android-pdf-viewer:2.8.2' |
After adding your build.gradle(Module:App) file will look like this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
apply plugin: 'com.android.application' android { compileSdkVersion 28 defaultConfig { applicationId "com.forogh.parwiz.pdfdocs" minSdkVersion 19 targetSdkVersion 28 versionCode 1 versionName "1.0" testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" } buildTypes { release { minifyEnabled false proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro' } } } dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'com.android.support:appcompat-v7:28.0.0-alpha1' implementation 'com.android.support.constraint:constraint-layout:1.1.0' implementation 'com.github.barteksc:android-pdf-viewer:2.8.2' testImplementation 'junit:junit:4.12' androidTestImplementation 'com.android.support.test:runner:1.0.2' androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2' } |
So now open your main_activity.xml and add this code, basically in here we are going to create a PDFView, and we have used the width and height as match_parent.
1 2 3 4 5 6 |
<com.github.barteksc.pdfviewer.PDFView android:layout_height="match_parent" android:layout_width="match_parent" android:id="@+id/pdfViewer" /> |
After adding of the PDFView, our activity_main.xml file looks like this, you can see that we are using ConstraintLayout for this purpose.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <com.github.barteksc.pdfviewer.PDFView android:layout_height="match_parent" android:layout_width="match_parent" android:id="@+id/pdfViewer" /> </android.support.constraint.ConstraintLayout> |
Now open your MainActivity.java first create the object of PDFView and after that add this code. in this code first we have created the object of our PDFView class, and after that we have found the id of PDFView in the xml file, also you can see that we have added some features for the PDFViewer.
1 2 3 4 5 6 7 8 |
private PDFView pdfview; pdfview = findViewById(R.id.pdfViewer); pdfview.useBestQuality(true); pdfview.enableSwipe(true); pdfview.fitToWidth(); pdfview.fromAsset("androidbook.pdf").load(); |
After adding the code will MainActivity.java look like this
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
package com.forogh.parwiz.pdfdocs; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import com.github.barteksc.pdfviewer.PDFView; public class MainActivity extends AppCompatActivity { private PDFView pdfview; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); pdfview = findViewById(R.id.pdfViewer); pdfview.useBestQuality(true); pdfview.enableSwipe(true); pdfview.fitToWidth(); pdfview.fromAsset("androidbook.pdf").load(); } } |
So now run the complete project and this will be the result
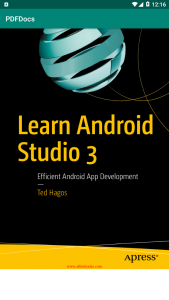
Watch the complete video tutorial for this article
Subscribe and Get Free Video Courses & Articles in your Email