In this Numpy tutorial we want to learn about Advance Python Numpy Techniques, for Numpy we can say Numerical Python, so Numpy is a powerful library in Python, and it is used for scientific computing and data analysis tasks. even though you will be familiar with the basics of Numpy, but there are some advanced techniques that you can use in Numpy, now let’s talk about these techniques.
Numpy Broadcasting
Broadcasting is powerful concept in Numpy and it allows arrays of different shapes to be used together in operations. It eliminates the need for explicit loops and enables efficient element computations. Broadcasting automatically aligns dimensions of different arrays, and it enables them to perform operations without explicitly matching their shapes.
1 2 3 4 5 6 7 8 9 |
import numpy as np # Create an array a = np.array([1, 2, 3]) # Perform broadcasting result = a + 5 print(result) |
In the above example, we have added scalar value 5 to an array using broadcasting. Numpy automatically aligns the dimensions, and it allows us to perform the addition without explicitly expanding the scalar to match the array shape.
This will be the result
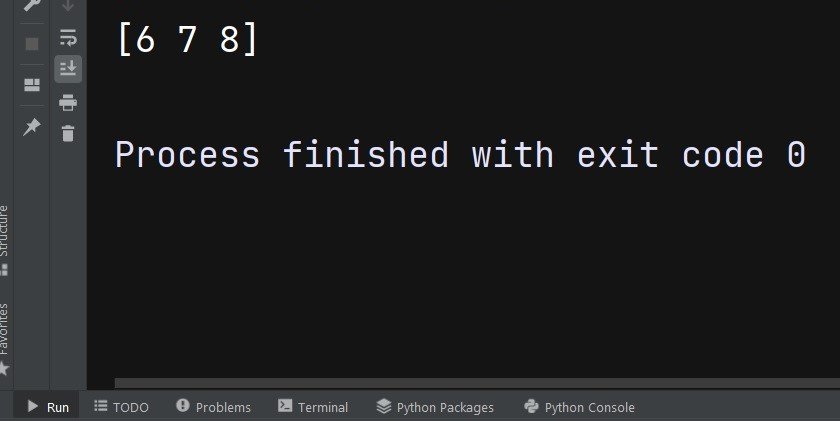
Numpy Universal Functions (ufuncs)
Numpy universal functions or ufuncs are another advanced feature that enables element operations on arrays. Ufuncs are highly optimized and it provide efficient execution of complex mathematical computations. Also they allows you to perform operations like exponentiation, logarithms, trigonometric functions and many more on entire arrays.
1 2 3 4 5 6 7 8 9 |
import numpy as np # Create an array a = np.array([1, 2, 3]) # Apply ufunc result = np.square(a) print(result) |
In the above example, we have used np.square() ufunc to calculate the square of each element in the array a. Ufuncs offers an efficient way to apply mathematical functions to arrays.
This is the output
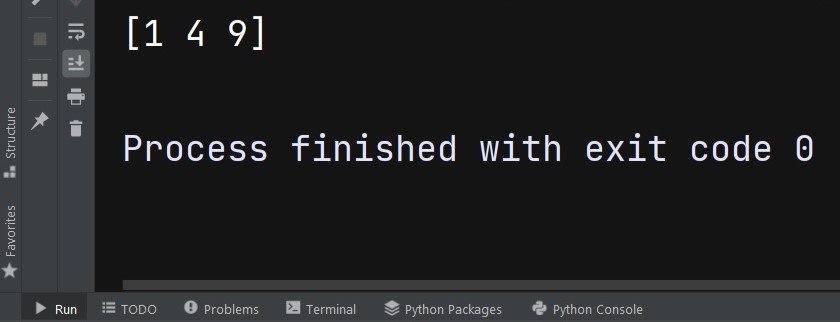
Numpy Array Manipulation
Numpy provides several advanced techniques for manipulating arrays, such as reshaping, stacking and splitting arrays.
a. Numpy Reshaping:
reshape() function allows you to change the shape of an array without modifying its data. This can be useful when you want to convert a 1D array into a 2D or 3D array, or vice versa.
1 2 3 4 5 6 7 8 9 |
import numpy as np # Create a 1D array a = np.array([1, 2, 3, 4, 5, 6]) # Reshape into 2D array b = a.reshape(2, 3) print(b) |
b. Numpy Stacking:
Numpy provides functions like np.concatenate(), np.vstack(), and np.hstack() for stacking arrays vertically and horizontally. These functions are particularly useful when you want to combine multiple arrays into a single array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
import numpy as np # Create arrays a = np.array([1, 2, 3]) b = np.array([4, 5, 6]) # Vertical stacking vstacked = np.vstack((a, b)) print(vstacked) # Horizontal stacking hstacked = np.hstack((a, b)) print(hstacked) |
c. Numpy Splitting:
The np.split() function allows you to split an array into multiple sub arrays along a specified axis. This can be useful when you want to divide a large array into smaller and manageable chunks.
1 2 3 4 5 6 7 8 9 |
import numpy as np # Create an array a = np.array([1, 2, 3, 4, 5, 6]) # Split into three sub arrays splitted = np.split(a, 3) print(splitted) |
Learn More on Python Numpy
- Python Numpy for Machine Learning
- How to Install Numpy
- Working with Linear Algebra in Numpy
- Numpy vs Pandas
Subscribe and Get Free Video Courses & Articles in your Email